Password hashing in a C# Windows app, absent ASP.NET's FormsAuthentication?
Solution 1
using System.Security.Cryptography;
public static string EncodePasswordToBase64(string password)
{ byte[] bytes = Encoding.Unicode.GetBytes(password);
byte[] inArray = HashAlgorithm.Create("SHA1").ComputeHash(bytes);
return Convert.ToBase64String(inArray);
}
Solution 2
The FormsAuthentication is defined in the System.Web.Security namespace which is in the System.Web.dll assembly.
Just because you are writing a WinForm app does not stop you from using that namespace or referencing that assembly; they are just not done by default as they would be for a WebForms app.
Solution 3
If you are using the hashing for user credentials I suggest you do more than just hashing, you ideally want key stretching as well.
Here is an API to do what you want in a secure fashion:
https://sourceforge.net/projects/pwdtknet/
Solution 4
Could you not use the BitConverter function instead of the "x2" loop?
e.g.
return BitConverter.ToString(hash).Replace("-", "");
Solution 5
I think it should work. All you need to do is reference System.Web.Security in your code (and add it as a reference in your Visual Studio Project).
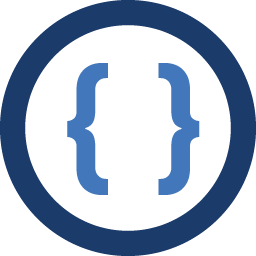
Admin
Updated on July 27, 2022Comments
-
Admin almost 2 years
My Win form app doesn't seem to like FormsAuthentication, I'm totally new to hashing so any help to convert this would be very welcome. Thanks.
//Write hash protected TextBox tbPassword; protected Literal liHashedPassword; { string strHashedPassword = FormsAuthentication.HashPasswordForStoringInConfigFile(tbPassword.Text, "sha1"); liHashedPassword.Text = "Hashed Password is: " + strHashedPassword; } //read hash string strUserInputtedHashedPassword = FormsAuthentication.HashPasswordForStoringInConfigFile( tbPassword.Text, "sha1"); if(strUserInputtedHashedPassword == GetUsersHashedPasswordUsingUserName(tbUserName.Text)) { // sign-in successful } else { // sign-in failed }
-
Andrew Shooner over 15 yearsOther than the obvious "it's not meant for WinForms apps", is there a reason why including System.Web.* is not such a good idea?
-
Kushal Shah about 15 yearsReminds me of a post by Rick Strahl at west-wind.com/Weblog/posts/617930.aspx 1. It doesn't "feel" right. 2. It forces System.Web into the loaded assebly list of any application consuming the library. 3. It adds 2.5 megs to the memory footprint just for loading it. 4. etc. (out of room)
-
Dan Esparza almost 14 yearsBear in mind that SHA1 is (apparently) faster, but would be less secure than SHA256. See en.wikipedia.org/wiki/SHA256 for more information
-
SLaks over 11 years@JarrodDixon: On the contrary. For passwords, you want a slower hash.
-
I. J. Kennedy over 11 yearsI don't see any source code there. Not sure anyone's going to trust their passwords to a .DLL they don't know anything about.
-
thashiznets over 10 yearsSource is there in the files area in the folder named "source". Source is provided for exactly the reason you describe. here is a link to the source folder sourceforge.net/projects/pwdtknet/files/Source