Perl - If string contains text?
Solution 1
If you just need to search for one string within another, use the index
function (or rindex
if you want to start scanning from the end of the string):
if (index($string, $substring) != -1) {
print "'$string' contains '$substring'\n";
}
To search a string for a pattern match, use the match operator m//
:
if ($string =~ m/pattern/) { # the initial m is optional if "/" is the delimiter
print "'$string' matches the pattern\n";
}
Solution 2
if ($string =~ m/something/) {
# Do work
}
Where something
is a regular expression.
Solution 3
For case-insensitive string search, use index
(or rindex
) in combination with fc
. This example expands on the answer by Eugene Yarmash:
use feature qw( fc );
my $str = "Abc";
my $substr = "aB";
print "found" if index( fc $str, fc $substr ) != -1;
# Prints: found
print "found" if rindex( fc $str, fc $substr ) != -1;
# Prints: found
$str = "Abc";
$substr = "bA";
print "found" if index( fc $str, fc $substr ) != -1;
# Prints nothing
print "found" if rindex( fc $str, fc $substr ) != -1;
# Prints nothing
Both index
and rindex
return -1
if the substring is not found.
And fc
returns a casefolded version of its string argument, and should be used here instead of the (more familiar) uc
or lc
. Remember to enable this function, for example with use feature qw( fc );
.
DETAILS:
From the fc
docs:
Casefolding is the process of mapping strings to a form where case differences are erased; comparing two strings in their casefolded form is effectively a way of asking if two strings are equal, regardless of case.
From the Unicode FAQ:
Q: What is the difference between case mapping and case folding?
A: Case mapping or case conversion is a process whereby strings are converted to a particular form—uppercase, lowercase, or titlecase—possibly for display to the user. Case folding is mostly used for caseless comparison of text, such as identifiers in a computer program, rather than actual text transformation. Case folding in Unicode is primarily based on the lowercase mapping, but includes additional changes to the source text to help make it language-insensitive and consistent. As a result, case-folded text should be used solely for internal processing and generally should not be stored or displayed to the end user.
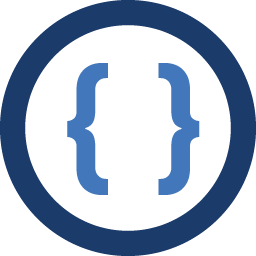
Admin
Updated on October 26, 2021Comments
-
Admin over 2 years
I want to use curl to view the source of a page and if that source contains a word that matches the string then it will execute a print. How would I do a
if $string contains
?In VB it would be like.
dim string1 as string = "1" If string1.contains("1") Then Code here... End If
Something similar to that but in Perl.
-
Eric Hartford almost 12 yearsbut what if "something" is in a variable?
-
Eugene Yarmash almost 12 years@Eric the
m//
operator interpolates variables. -
Dave Cross almost 12 yearsNo need for the match operator if what you're matching isn't a regular expression. For a plain text string the
index
function works just fine. -
Grace Huang over 11 yearsjust for a substring, you should use
index
, which is about 4x faster than regex. -
mabalenk almost 3 yearsCan you please clarify, what is a "casefolded version of a string argument"?
-
Timur Shtatland almost 3 years@mabalenk It is an operation done for case-insensitive string comparison. I added a clarification to the answer.