PermissionError: [Errno 13] Permission denied
Solution 1
This happens if you are trying to open a file, but your path is a folder.
This can happen easily by mistake.
To defend against that, use:
import os
path = r"my/path/to/file.txt"
assert os.path.isfile(path)
with open(path, "r") as f:
pass
The assertion will fail if the path is actually of a folder.
Solution 2
There are basically three main methods of achieving administrator execution
privileges on Windows.
- Running as admin from
cmd.exe
- Creating a shortcut to execute the file with elevated privileges
- Changing the permissions on the
python
executable (Not recommended)
A) Running cmd.exe
as and admin
Since in Windows there is no sudo
command you have to run the terminal (cmd.exe
) as an administrator to achieve to level of permissions equivalent to sudo
. You can do this two ways:
-
Manually
- Find
cmd.exe
inC:\Windows\system32
- Right-click on it
- Select
Run as Administrator
- It will then open the command prompt in the directory
C:\Windows\system32
- Travel to your project directory
- Run your program
- Find
-
Via key shortcuts
- Press the windows key (between
alt
andctrl
usually) +X
. - A small pop-up list containing various administrator tasks will appear.
- Select
Command Prompt (Admin)
- Travel to your project directory
- Run your program
- Press the windows key (between
By doing that you are running as Admin so this problem should not persist
B) Creating shortcut with elevated privileges
- Create a shortcut for
python.exe
- Righ-click the shortcut and select
Properties
- Change the shortcut target into something like
"C:\path_to\python.exe" C:\path_to\your_script.py"
- Click "advanced" in the property panel of the shortcut, and click the option "run as administrator"
Answer contributed by delphifirst in this question
C) Changing the permissions on the python
executable (Not recommended)
This is a possibility but I highly discourage you from doing so.
It just involves finding the python
executable and setting it to run as administrator every time. Can and probably will cause problems with things like file creation (they will be admin only) or possibly modules that require NOT being an admin to run.
Solution 3
Make sure the file you are trying to write is closed first.
Solution 4
Change the permissions of the directory you want to save to so that all users have read and write permissions.
Solution 5
You can run CMD as Administrator and change the permission of the directory using cacls.exe. For example:
cacls.exe c: /t /e /g everyone:F # means everyone can totally control the C: disc
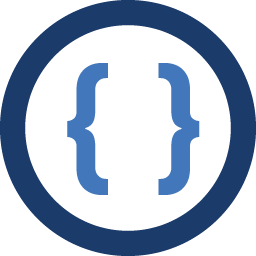
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I'm getting this error :
Exception in Tkinter callback Traceback (most recent call last): File "C:\Python34\lib\tkinter\__init__.py", line 1538, in __call__ return self.func(*args) File "C:/Users/Marc/Documents/Programmation/Python/Llamachat/Llamachat/Llamachat.py", line 32, in download with open(place_to_save, 'wb') as file: PermissionError: [Errno 13] Permission denied: '/goodbye.txt'
When running this :
def download(): # get selected line index index = films_list.curselection()[0] # get the line's text selected_text = films_list.get(index) directory = filedialog.askdirectory(parent=root, title="Choose where to save your movie") place_to_save = directory + '/' + selected_text print(directory, selected_text, place_to_save) with open(place_to_save, 'wb') as file: connect.retrbinary('RETR ' + selected_text, file.write) tk.messagebox.showwarning('File downloaded', 'Your movie has been successfully downloaded!' '\nAnd saved where you asked us to save it!!')
Can someone tell me what I am doing wrong?
Specs : Python 3.4.4 x86 Windows 10 x64
-
MarshallMa almost 6 yearsThanks. It works when I run anaconda prompt as admin.
-
Gulzar almost 4 yearsWhat if this hapens from PyCharm? I am unable to give admin privilages to python.exe because this is a work computer.
-
Mixone almost 4 yearsIn that case you probably need to contact your IT support team. Or just move the file creation/deletion to a directory you have write access on your work pc
-
Mixone almost 4 yearsAlso try running PyCharm as an Admin, if you can, not the python.exe
-
Gulzar about 3 yearsor you could just use
path = os.path.abspath(path)
-
Gulzar about 3 yearsI was going to edit your namings to be cohesive, then noticed you got the error for
folder_path
, and didn't get the error forimg_path
. This makes me believe Keras isn't bugged, and the real solution to your problem is stackoverflow.com/a/62244490/913098 -
Neil_UK about 3 years@Mixone Why does the OP need admin privileges? If he owns the destination folder, and owns the program, then surely the levels match? How does one debug what level you're running at, and what level you need for any specific destination?
-
d33tah over 2 yearsWARNING: the first two snippets here have a vulnerability called OS command injection: whitehatsec.com/glossary/content/os-command-injection ; always use subprocess and never combine shell=True or os.system and user-controlled variables.
-
Admin over 2 yearsPlease add further details to expand on your answer, such as working code or documentation citations.
-
Phenyl over 2 yearsIndeed, if the file is open in Excel, you cannot
open
it. -
Nesha25 over 2 yearsI wish the original asker would accept this as the answer. There are many, many questions about this error, and this is the only answer that really seems to be right. Often this error occurs despite the file having correct permissions set, and so the answer has to be something else. This is it.
-
Arun Sai Mustyala over 2 yearsI'm running pydev project in windows and this solution worked fine for me
-
Gulzar over 2 yearsAlso, instead of all the "\\", you can (and should) use
os.path.sep
, or better, useos.path.join
-
Mixone about 2 years@Neil_UK I beleive it was due to the fact that their code allows the user to CHOOSE a directory to save in also some work and school pcs have a lot of gpo policies blocking write access to some folders that are sometimes shared, he needs the priv if he doesnt own it is the point, you know the level in microsoft due to your user