PG::UndefinedColumn: ERROR: column comments.post_id does not exist
Solution 1
Yes, you're missing the field post_id
on the Comment
model, which would tell Rails which comments belong to which posts.
You can add it by generating a migration from the command line like so:
rails generate migration AddPostIdToComments post:references
rake db:migrate
Solution 2
You probably just need to add the column into your comments
table.
You can do that by creating a migration via the terminal to add the column in:
$ rails g migration add_post_id_column_to_comments post:belongs_to
$ rake db:migrate
The reason you want to use post:belongs_to
is that Rails will automatically append the _id
suffix and create a foreign key in the comments table to refer back to each other.
So essentially this part of the migration post:belongs_to
will add the column post_id
to your comments table. (Same thing if you did for example cars:belongs_to
, you would get cars_id
, etc)
That way you can be able to refer to the post's comments like this:
@post.comments
The reason why your @post.comments
is failing now is that it is looking for that post_id
column that you have not made yet which is probably also because you may not have defined the relationship between your Post
and Comment
models.
If you haven't done so already you just need to quickly define the relationship in each model:
- A post has many comments.
- A comment
belongs to
a post.
Get the lingo?
In your Post
model, just add
class Post < ActiveRecord::Base
has_many :comments # make sure it's pluralized
end
and in your Comment
model, add
class Comment < ActiveRecord::Base
belongs_to :post # and this one is singularized
end
Then try running your app again. Let me know how it goes.
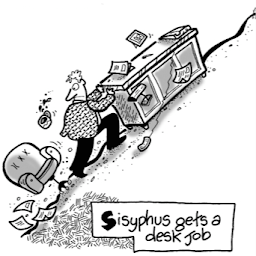
Isaac Byrne
I am an Austin-based developer. If I'm not developing websites or applications for clients, I usually find myself exploring datasets and experimenting with machine learning. I also love financial markets, specifically the futures and forex market. I have a diverse skill set ranging from design, to front-end and back-end development, all the way to data science and machine learning. I am experienced working with React, Node.Js, MongoDB, Meteor, Sass, Gulp, Git, and Python.
Updated on June 07, 2022Comments
-
Isaac Byrne almost 2 years
I cant seem to figure out why I keep getting this error. I am trying to add comment forms to a blog I am making and it wont work. Here is what the full error says.
ActiveRecord::StatementInvalid in Posts#show
Showing /Users/ipbyrne/FirstRailsApp/blog/app/views/posts/show.html.erb where line #17 raised:
PG::UndefinedColumn: ERROR: column comments.post_id does not exist LINE 1: SELECT COUNT() FROM "comments" WHERE "comments"."post_id" ... ^ : SELECT COUNT() FROM "comments" WHERE "comments"."post_id" = $1
</p> <div id="comments"> <h2><%= @post.comments.count %> Comments</h2> <-- Says it breaks here <%= render @post.comments %> <h3>Add a comment:</h3>
From what i can understand there is a column missing in one of the tables in the schema.rb file? Incase this the case here is what mine looks like
# encoding: UTF-8 # This file is auto-generated from the current state of the database. Instead # of editing this file, please use the migrations feature of Active Record to # incrementally modify your database, and then regenerate this schema definition. # # Note that this schema.rb definition is the authoritative source for your # database schema. If you need to create the application database on another # system, you should be using db:schema:load, not running all the migrations # from scratch. The latter is a flawed and unsustainable approach (the more migrations # you'll amass, the slower it'll run and the greater likelihood for issues). # # It's strongly recommended that you check this file into your version control system. ActiveRecord::Schema.define(version: 20140911230918) do create_table "comments", force: true do |t| t.string "name" t.text "body" t.integer "post_id" t.datetime "created_at" t.datetime "updated_at" end add_index "comments", ["post_id"], name: "index_comments_on_post_id" create_table "posts", force: true do |t| t.string "title" t.text "body" t.datetime "created_at" t.datetime "updated_at" end create_table "users", force: true do |t| t.string "email", default: "", null: false t.string "encrypted_password", default: "", null: false t.string "reset_password_token" t.datetime "reset_password_sent_at" t.datetime "remember_created_at" t.integer "sign_in_count", default: 0, null: false t.datetime "current_sign_in_at" t.datetime "last_sign_in_at" t.string "current_sign_in_ip" t.string "last_sign_in_ip" t.datetime "created_at" t.datetime "updated_at" end add_index "users", ["email"], name: "index_users_on_email", unique: true add_index "users", ["reset_password_token"], name: "index_users_on_reset_password_token", unique: true end