PHP 5 SOAP client returns NULL when WSDL-provided function is called
Solution 1
I found this whole thing pretty confusing when I started using the SoapClient libraries in php. The proper way to format this stuff is as follows:
$client = new SoapClient( $wsdl_url, array( "trace" => 1 ) );
$params = array(
"sharedSecret" => "thisIsSomeSecret",
"searchParams" => "thisIsSomeSearchParam"
);
$response = $client->basicSearch( $params );
Without seeing the response, I can't tell you how to reference the return parameters, but the way its stored is as a member var of a stdClass object. Such that you reference the returns like this...
$reponse->paramName;
A nice trick if you keep the array( "trace" => 1 ) set of options in there is that you can call these two functions....
$respXML = $client->__getLastResponse();
$requXML = $client->__getLastRequest();
... to see the actual xml that gets sent out, to see if its well-formed. Careful, SoapClient is pretty buggy. Much better than nusoap though, don't go for that trash.
Solution 2
yeap
$client = new SoapClient( $wsdl_url, array( "trace" => 1 ) );
"trace" parameter helps
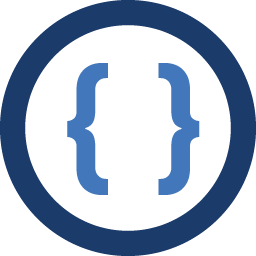
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
I am trying to use a webservice to look for specific users from my PHP application. I have used that exact same webservice in ASP.NET with success.
Basically, I do everything like the PHP doc tells me to, and I use the same methods/variables I used in ASP.NET (for the webservice itself), but I can't seem to get a result.
The function is listed in __getFunctions() and should return
anyType
, which if I understand correctly is the equivalent ofmixed
in PHP:array(1) { [0]=> string(63) "anyType basicSearch(string $sharedSecret, string $searchParams)" }
When I do call basicSearch() though, it seems returns NULL.
basicSearch() is supposed to return an XML document with the information. In ASP.NET I used to simply cast the response to, I believe, and XmlDocument. Should I do that in PHP too? With which representation of an XML document (SimpleXML, DOM, etc.)?
Could it show as NULL just because PHP can't understand the format?
Am I doing something wrong in PHP? Or should I look into the webservice itself and try to debug on that side?
<?php $client = new SoapClient($wsdl_url); echo $client->__getFunctions(); echo "<br />\n"; echo $client->basicSearch($key, $req); ?>
PS: I am using the PHP 5 library. Maybe using some other library like nu-soap would help? There seems to be more doc online about it.
Update:
Using an array to pass the parameter does not work, SOAP expects separate arguments. Calling __getLastRequest() returns a string concatenating $key and $req with no other XML. Calling __getLastResponse() returns an empty string. No exception is thrown whatsoever. It seems PHP does not know what to do with the arguments I give it, even though it has parsed the WSDL file since I get the function I use listed when I call __getFunctions().
Any help would be appreciated.
Update': Still no solution working. I am baffled...
-
Henrik Opel over 14 yearsI disagree on the notation/format remark: You pass the arguments as an array only if you'd use the
$client->__soapCall($functionName, $arguments)
mehtod, which is mainly intended for non WSDL usage. If you use WSDL, you should call functions directly by name, passing the arguments separately, as the OP does. -
Henrik Opel over 14 yearsBut I agree on using the trace option and __getLastRequest() to check for the actual returned value.
-
Admin over 14 yearsAs Henrik Opel mentionned, the array method does not seem to work. PHP complains about a lacking parameter that's required. When I use trace and __getLastRequest() and __getLastResponse() I get a concatenation of the first parameter and the second as my request and an empty string as a response, as if PHP did not know what to do with my request. A call to __getFunctions() returns the function I use though, so the WSDL is read... This whole thing is confusing.
-
Josh over 14 yearsI found where my differences come in -- depending on how your wsdl is architected and what language/package is publishing the soap service endpoint, you can get into a situation where the parameters are expected as an array/object. The soap services I work with at my job are published by EJB's - they are returning stdObjects and expect the parameters to be passed in as arrays rather than sequentially.... anyway, just thought I'd follow up.