PHP array into google charts
i see you haven't found out how to do it after your first question, so i've made you a working example here, hope this helps you Dave :).
If you've got some questions about this, feel free to ask!
<?php
//create array variable
$values = [];
//pushing some variables to the array so we can output something in this example.
array_push($values, array("year" => "2013", "newbalance" => "50"));
array_push($values, array("year" => "2014", "newbalance" => "90"));
array_push($values, array("year" => "2015", "newbalance" => "120"));
//counting the length of the array
$countArrayLength = count($values);
?>
<script type="text/javascript" src="https://www.google.com/jsapi"></script>
<script type="text/javascript">
google.load("visualization", "1", {packages:["corechart"]});
google.setOnLoadCallback(drawChart);
function drawChart() {
var data = new google.visualization.DataTable();
data.addColumn('string', 'Year');
data.addColumn('number', 'Balance');
data.addRows([
<?php
for($i=0;$i<$countArrayLength;$i++){
echo "['" . $values[$i]['year'] . "'," . $values[$i]['newbalance'] . "],";
}
?>
]);
var options = {
title: 'My Savings',
curveType: 'function',
legend: { position: 'bottom' }
};
var chart = new google.visualization.LineChart(document.getElementById('curve_chart'));
chart.draw(data, options);
}
</script>
<div class="grid-container">
<div class="grid-100 grid-parent">
<div id="curve_chart" style="width: 100%; height: auto"></div>
</div>
</div>
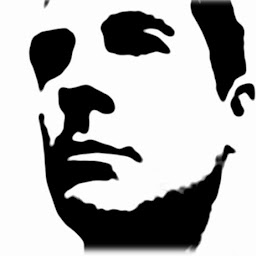
Dave
Updated on June 14, 2022Comments
-
Dave almost 2 years
I need a little help putting PHP data into google charts.
I have created a simple array
$chart_arr = array($year, $new_balance); json_encode($chart_arr);
If I run
<?php echo json_encode($chart_arr);?>
I see the following: [2015,1150] [2016,1304.5] [2017,1463.635] [2018,1627.54405] [2019,1796.3703715], so I think (?) I am getting the right numbers encoded from my forloop that generates $year and $new_balance.
I want to chart these numbers in google chart
<script type="text/javascript"> google.load("visualization", "1", {packages:["corechart"]}); google.setOnLoadCallback(drawChart); function drawChart() { var data = new google.visualization.DataTable(); data.addColumn('string', 'Year'); data.addColumn('number', 'Balance'); data.addRows([ <?php echo json_encode($chart_arr);?> ]);
Or alternatively:
data.addRows([ <?php echo $chart_arr;?> ]);
And then continues...
var options = { title: 'My Savings', curveType: 'function', legend: { position: 'bottom' } }; var chart = new google.visualization.LineChart(document.getElementById('curve_chart')); chart.draw(data, options);
}
displaying as...
<div class="grid-container"> <div class="grid-100 grid-parent"> <div id="curve_chart" style="width: 100%; height: auto"></div> </div> </div>
I have tried a number of variations but am either not getting the data into the chart or not getting a chart to display.
Could someone help me to show where I am going wrong?
I saw another related post that used the following code:
$chartsdata[$i] = array($testTime, $testNb); echo json_encode($chartsdata); var jsonData = $.ajax({ url: "test.php", dataType: "json", async: false }).responseText; var obj = JSON.stringify(jsonData); data.addRows(obj);
Is this the approach I need to be looking at?
Thanks in advance
-
Dave over 9 yearsThanks Koen. I couldn't get past the issue that all I charted was the ultimate value of $year and $newbalance for each year rather than the one corresponding to that year. I'll try this out. Thanks again.
-
Dave over 9 yearsThanks Joel, I'm looking at these 2 suggestions. Could you clarify what should go with the data field? Secondly is this the correct way to represent the JSON data $chart_arr = array($year, $new_balance); json_encode($chart_arr); - thanks
-
Dave over 9 yearsKoen, Thanks again. Your code works fine. I guess the issue is that I don't understand enough so when trying to incorporate my $chart_arr data into it it fails and gives me no explanation why. I think I'll have to drop it as I've wasted too much time to achieve nothing. Thanks again for your help.
-
Joel Lubrano over 9 yearsThe data option can be thought of as anything you might submit in an HTTP form. You can use JQuery's serialize method if you have a form with data on a page. As an example, suppose you allowed your user to select a date range for the data that will be graphed. The data field could be
startDate=2015-01-01&endDate=2015-01-14
. You could also create a Javascript object and use JSON.stringify. There are a lot of options available. If you really are seeing[2015,1150], [2016,1304.5], ...
from your json_encode call then you do seem to be formatting your $chart_arr correctly. -
Dave over 9 yearsthe issue is that the $chart_arr data is not feeding into the google api. All I see is a chart with no data and a vertical scale from 0 to 1. What part of this function picks up the array data?
-
Joel Lubrano over 9 yearsThe success function in the AJAX call should pick up the data then add it to your Google DataTable. If you
console.log(json)
andconsole.log(jsonData)
in the success function, what do you see? -
Dave over 9 yearsFailed to load resource: net::ERR_CACHE_MISS
-
Joel Lubrano over 9 yearsThat error message is actually from a bug in Chrome. Let's continue this discussion in chat.
-
Dave over 9 yearsit appears that I don't have a sufficient reputation to use the chat function :( Somewhere else I can contact you?
-
Joel Lubrano over 9 yearsI am working on a jsfiddle for you that should help. Give me one sec.
-
Dave over 9 yearsHi Joel, thanks for that. Sorry for delay but only just catching up now. Do i need to swap the var data to be = { json: JSON.stringify(['chart_arr']) }; in my example to use the $chart_arr array i have created? Secondly what goes in the url field? Should that be the complete url to this file? Thanks