php array_search 0 index
Solution 1
This is explicitly mentioned in the docs. You need to use ===
or !==
:
$key = array_search(...);
if ($key !== false) ...
Otherwise, when $key
is 0
, which evaluates to false
when tested as a boolean.
Solution 2
The conditional in your second example block gives execution order priority to the !==
operator, you want to do the opposite though.
if (($key = array_search(100,$test)) !== false) {
!==
has higher precedence than ==
which makes the parentheses necessary.
Solution 3
$key = array_search($what, $array);
if($key !== false and $array[$key] == $what) {
return true;
}
it's more secure
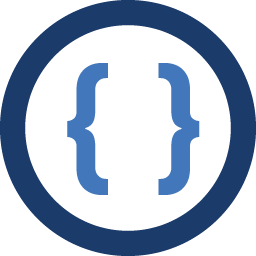
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
It seems that that you can not use the search_array function in PHP to search the 0 index AND have it evalute as true.
Consider this code for example:
$test=array(100, 101, 102, 103); if($key=array_search(100,$test)){ echo $key; } else{ echo "Not found"; }
The needle '100' is found in the haystack and the key is returned as 0. So far so good, but then when I evaluate whether the search was successful or not it fails because the returned value is 0, equal to false!
The php manual suggests using '!==' but by doing so the key (array index) is not returned, instead either 1 or 0 is returned:
if($key=(array_search(103,$test)!== false)){ }
So how can I successfully search the array, find a match in the 0 index and have it evaluate as true?