PHP dynamically create CSV: Skip the first line of a CSV file
Solution 1
As you are keeping track of the row number anyway, you can use continue
to skip the rest of the loop for the first row.
For example, add this at the start of your while loop (just above $num = count($data)
):
if($row == 1){ $row++; continue; }
There are other ways to do this, but just make sure that when you continue, $row
is still being incremented or you'll get an infinite loop!
Solution 2
Rather than using if condition for checking whether it is the first row, a better solution is to just add an extra line of code before the line from where the while loop starts as shown below :
....
.....
fgetcsv($handle);//Adding this line will skip the reading of th first line from the csv file and the reading process will begin from the second line onwards
while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) {
.......
.......
It is just as simple......
Solution 3
put this inside your while loop:
if ($row == 1) continue;
Solution 4
Please use the following lines of code
$flag = true;
while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) {
if($flag) { $flag = false; continue; }
//your code for insert
}
Having the flag variable as true and setting it to false will skip the first line of the CSV file. This is simple and easy to implement.
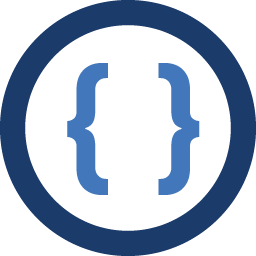
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I am trying to import a CSV file. Due to the program we use, the first row is basically all headers that I would like to skip since I've already put my own headers in via HTML. How can I get the code to skip the first row of the CSV? (the strpos command is to cut off the first field in all the rows.)
<?php $row = 1; if (($handle = fopen("ptt.csv", "r")) !== FALSE) { while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { $num = count($data); $row++; for ($c=0; $c < $num; $c++) { if(strpos($data[$c], 'Finished') !== false) { $c++; echo "<TR> <TD nowrap>" . $data[$c] . "</ TD>"; } Else{ echo "<TD nowrap>" . $data[$c] . "</ TD>"; } } } fclose($handle); } ?>