PHP how to save HTML string into database
Solution 1
I use base64 encoded data to store in my Database with the BLOB datatype. The boilerplate code is as follow.
$content = '<html>
<head>
<script>--Some javascript and libraries included--</script>
<title></title>
</head>
<body>
<style>--Some Styling--</style>
</body>
</html>';
To encode data in base64
$encodedContent = base64_encode($content); // This will Encode
And save the data in database with BLOB. Now after retrieve data, just decode it as follow.
$ContentDecoded = base64_decode($content); // decode the base64
Now the value of $contentDecoded is the plain HTML.
Solution 2
If you base64 encode it you increase the storage size by rougly 30% and you need to decode it each time you display it. Look at the table structure for Wordpress, the most widely used software that stores html on a mysql database using php. What do they use? LONGTEXT. In your case TEXT is probably better because you probably have a good idea about the size of the page.
Solution 3
Store HTML into a variable using addslashes()
function.
$html = addslashes('<div id="intro">
<div id="about" align="left">
<h2 class="bigHeader" dir="rtl"HEADER</h2>
<img src="img/Med-logo.png" alt="" />
<div id="wellcomePage" class="text-left text" dir="rtl">
<p>...some words....</p>
<p>.some words....</p>
<p> </p>
</div>
</div>
</div>');
After this, form an SQL query.
$sql = "UPDATE `Pages` SET `content`= '".$html."'";
and you have to add stripslashes
when retrieve from DB
Solution 4
I would recommend you to use TEXT
. Blobs are typically used to store images, audio or other multimedia objects. read more about bolobs
Data type to store HTML in Database would be TEXT
.
Use mysql_real_escape_string() to store html text in database
$content = '<html>
<head>
<script>--Some javascript and libraries included--</script>
<title></title>
</head>
<body>
<style>--Some Styling--</style>
</body>
</html>';
$html = mysql_real_escape_string($content);
Solution 5
you can use base64_encode and store that string into db with text/blob type of field
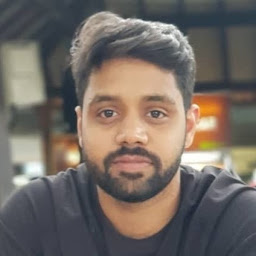
Comments
-
saiid almost 2 years
In response to an API call, I'm getting a full HTML script. Full means it includes HTML CSS and Javascript. Now I have that HTML as string in PHP variable.
$content = '<html> <head> <script>--Some javascript and libraries included--</script> <title></title> </head> <body> <style>--Some Styling--</style> </body> </html>';
Now, what is the best way to save this variable in Database and How?
- As a string with VARCHAR or TEXT type?
- As a string with Base64 Encoded with VARCHAR or TEXT type?
- As a Binary with BLOB type?
Or any other you would like to suggest(May be Serialize or Pack)?