PHP json_decode return empty array
Solution 1
you should not use echo because it is an array. use print_r or var_dump .it works fine
$json = '{"a":1,"b":2,"c":3,"d":4,"e":5}';
print_r(json_decode($json, true));
Output:
Array
(
[a] => 1
[b] => 2
[c] => 3
[d] => 4
[e] => 5
)
Solution 2
You can validate at following website: http://jsonlint.com/
You have to use a php "json_decode()" function to decode a json encoded data. Basically json_decode() function converts JSON data to a PHP array.
Syntax: json_decode( data, dataTypeBoolean, depth, options )
data : - The json data that you want to decode in PHP.
dataTypeBoolean(Optional) :- boolean that makes the function return a PHP Associative Array if set to "true", or return a PHP stdClass object if you omit this parameter or set it to "false". Both data types can be accessed like an array and use array based PHP loops for parsing.
depth :- Optional recursion limit. Use an integer as the value for this parameter.
options :- Optional JSON_BIGINT_AS_STRING parameter.
Now Comes to your Code
$json_string = '{"a":1,"b":2,"c":3,"d":4,"e":5}' ;
Assign a valid json data to a variable $json_string within single quot's ('') as json string already have double quots.
// here i am decoding a json string by using a php 'json_decode' function, as mentioned above & passing a true parameter to get a PHP associative array otherwise it will bydefault return a PHP std class objecy array.
$json_decoded_data = json_decode($json_string, true);
// just can check here your encoded array data.
// echo '<pre>';
// print_r($json_decoded_data);
// loop to extract data from an array
foreach ($json_decoded_data as $key => $value) {
echo "$key | $value <br/>";
}
Solution 3
It works fine, but you use wrong method to display array.
To display array you cannot use echo
but you need to use var_dump
Solution 4
It works fine as others mention, but when you print the array it is converted to string, which means only the string "Array" will be printed instead of the real array data. You should use print_r()
, var_dump()
, var_export() or something similar to debug arrays like this.
If you turn on notices you will see:
PHP Notice: Array to string conversion in ...
The example you linked uses also var_dump for the same reason.
Solution 5
No, it doesn't return an empty array.
Printing an array with echo
just prints a string "Array()"
.
Use print_r
or var_dump
to get the structure of the variable.
In newer PHP it will also emit a notice when using echo
on an array ("Array to string conversion"), so you shouldn't do it anyway. The manual you've mentioned changed to print_r
.
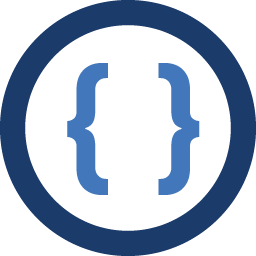
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I just test this sample from php doc (http://au2.php.net/manual/en/function.json-decode.php)
here is my code:
<?php $json = '{"a":1,"b":2,"c":3,"d":4,"e":5}'; echo json_decode($json, true), '<br />';?>
But it just returns an EMPTY array.
Have no idea why...Been searching around but no solution found.
PLEASE help!
-
Sheikh Abdul Wahid over 7 yearsit making empty object {} as empty array []. but your instruction to provide second argument as true it really works. so 1+ from me.