PHP return type
Solution 1
As per this page : http://php.net/manual/en/language.types.type-juggling.php
You can try doing,
return (boolean) $value;
Now PHP offer return type declaration from PHP 7. I have explained how to use return type in PHP
Solution 2
Since this question still comes up in search engine results, here is an up-to-date answer:
PHP 7 actually introduced proper return types for functions / methods as per this RFC.
Here is the example from the manual linked above:
function sum($a, $b): float {
return $a + $b;
}
Or, in a more general notation:
function function_name(): return_type {
// some code
return $var // Has to be of type `return_type`
}
If the returned variable or value does not match the return type, PHP will implicitly convert it to that type. Alternatively, you can enable strict typing for the file via declare(strict_types=1);
, in which case a type mismatch will lead to a TypeError Exception.
Easy as that. However, remember that you need to make sure that PHP 7 is available on both, your development and production server.
Solution 3
It's not possible to explicitly define the return type at the method/function level. As specified, you can cast in the return, so for example ...
return (bool)$value;
Alternatively, you can add a comment in phpDoc syntax, and many IDEs will pick up the type from a type completion perspective.
/**
* example of basic @return usage
* @return myObject
*/
function fred()
{
return new myObject();
}
Solution 4
Return type added in PHP 7 ;)
https://wiki.php.net/rfc/return_types
Solution 5
Since PHP 7.0, you can declare the return type like this:
function returnABool(): bool {
return false;
}
Not only common data types, you can return expected object instances as well. For example you expect a function to always return an instance of a class. This can be achieved like this:
class Biography {
public function name() {
return 'John Doe';
}
}
Now you can declare the return type for this object like this:
function get_bio(): Biography {
return new Biography();
}
When the function or method returns an unexpected data type, a TypeError is thrown. Here is an example where you will encounter a TypeError.
class Biography {
public function name() {
return 'John Doe';
}
}
class Bio2 {
public function name() {
return 'John Doe';
}
}
function get_bio(): Biography {
return new Bio2;
}
And an example that will not throw any TypeError:
class Biography {
public function name() {
return 'John Doe';
}
}
class Bio2 {
public function name() {
return new Biography();
}
}
function get_bio(): Biography {
$bio2 = new Bio2;
return $bio2->name();
}
More details on declaring return data types for functions and methods can be learnt from the official documentation.
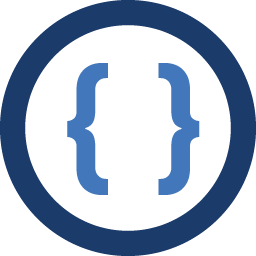
Admin
Updated on June 15, 2021Comments
-
Admin almost 3 years
Is it possible to define the return type like this?
public static function bool Test($value) { return $value; //this value will be bool }