PHP search MySQL table
Solution 1
Non-numeric field must be enclosed with single quotes in a query.
<?php
$search = $_POST["search"];
mysql_connect("localhost", "username", "password") OR die (mysql_error());
mysql_select_db ("your_db_name") or die(mysql_error());
$query = "SELECT * FROM `profile` WHERE `email`='$search'";
$result = mysql_query($query) or die (mysql_error());
if($result)
{
while($row=mysql_fetch_row($result))
{
echo $row[0],$row[1],$row[2];
}
}
else
{
echo "No result";
}
?>
<form action="profile.php" method="post">
<input type="text" name="search"><br>
<input type="submit">
</form>
EDIT:
In above code-snippet you have single text
field and a submit button
so you can use "search"
field value to search on any one database field at a time.
If you want to search on email then sql query will be:
SELECT * FROM `profile` WHERE `email`='$search'";
You can use OR
operator to search on one or more field:
SELECT * FROM `profile` WHERE `email`='$search' or about`='$search'";
You can use LIKE operator to search a string
SELECT * FROM `profile` WHERE `email` LIKE '%$search%'";
Solution 2
Simplest way is write another query
$query = "SELECT * FROM profile WHERE email='$email'";
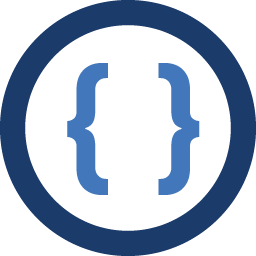
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I've built this little script that searches a table in my database, but for the life of me I can't seem to find anywhere on how to display the table information if someone only searches for the email. I want to be able to search for only the email and have it display all the information for that email address from the database. Any thoughts?
<?php $profile = $_POST["profile"]; mysql_connect ("", "", ""); mysql_select_db (""); $query = "SELECT * FROM `profile` WHERE `email`='$email' and `about`='$about' and `age`='$age' and `sex`='$sex' and `website`='$website'"; $result = mysql_query ($query); if ($result) { while($row=mysql_fetch_row($result)) { echo $row[0],$row[1],$row[2]; } }else{ } ?> <form action="profile.php" method="post"> <input type="text" name="search"><br> <input type="submit">
-
Admin over 12 yearsWill this display the result or is it the best way the get the $query?
-
KV Prajapati over 12 years@Pxlc - Yes! it will display the result. See I've edit my post.
-
Admin over 12 yearsAre you sure that works? It's not working on my end, try [email protected] arnid.us/profile.php
-
KV Prajapati over 12 years@Pxlc - Please post the code in the opening post that you have in given link.
-
Admin over 12 yearsI'm still not seeing any results. I'm using Heroku as a host, but that shouldn't cause any backend problems. I replaced the original SELECT with SELECT * FROM
profile
WHEREemail
='$profile'"; -
KV Prajapati over 12 years@Pxlc - Have a look at edited post. There is a problem with $_POST["profile"] because "profile" isn't in request collection. Use $_POST["search"].
-
Admin over 12 yearsIt worked :) Is there any way to make the results display in HTML rather than echo. Could I just do <p>$row[0]</p> ?
-
KV Prajapati over 12 years@Pxlc - Yes, you can write html tags too along with result. echo "<p>$row[0]</p>";
-
Admin over 12 yearsOkay, awesome thanks dude :) Out of curiosity, could arnid.us/[email protected] display results as well?