PHP SoapClient request
There are two possible Params subscriber and overwriteable
<soap:Body>
<AddSubscriber xmlns="admin.ekeryx.com">
<subscriber>
<ID>string</ID>
<FirstName>string</FirstName>
<LastName>string</LastName>
<Email>string</Email>
</subscriber>
<overwritable>boolean</overwritable>
</AddSubscriber>
So you need to do some more complex construction by using SoapVar to represent the subsrciber structure.
http://www.php.net/manual/en/soapvar.soapvar.php
Should look something like this i think, although youll want to check the XSD against the Soap:Body produced...
$subscriber = new StdClass();
$subscriber->ID = 'myid';
$subscriber->FirstName = 'First';
$subscriber->LastName = 'Last';
$subscriber = new SoapParam(new SoapVar($subscriber, SOAP_ENC_OBJECT, $type, $xsd), 'subscriber');
$type should be the type in the XSD/WSDL defeinition for the api and $xsd is the URI for the XSD.
I think that should do it but Ive only used native PHP libs once for EBay (it was a nightmare haha) and that was almost 2 years ago so im a little rusty.
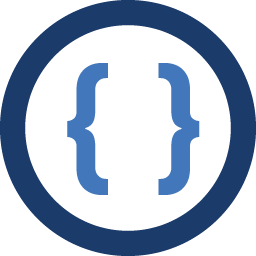
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm trying to send a SOAP request to a newsletter service using this WSDL.
Here's my PHP:
$client = new SoapClient($wsdl_url, array( 'login' => 'myusername', 'password' => 'mypassword', 'trace' => true )); $client->AddSubscriber( new SoapParam('MyFirstName', 'FirstName'), new SoapParam('MyLastName', 'LastName'), new SoapParam('[email protected]', 'Email') );
I'm getting the exception:
End element 'Body' from namespace 'schemas.xmlsoap.org/soap/envelope/' expected. Found element 'LastName' from namespace ''. Line 2, position 156.
Here's what the service is expecting for AddSubscriber:
<?xml version="1.0" encoding="utf-8"?> <soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="schemas.xmlsoap.org/soap/envelope/"> <soap:Header> <AuthHeader xmlns="admin.ekeryx.com"> <Username>string</Username> <Password>string</Password> <AccountID>string</AccountID> </AuthHeader> </soap:Header> <soap:Body> <AddSubscriber xmlns="admin.ekeryx.com"> <subscriber> <ID>string</ID> <FirstName>string</FirstName> <LastName>string</LastName> <Email>string</Email> </subscriber> <overwritable>boolean</overwritable> </AddSubscriber> </soap:Body> </soap:Envelope>
Here's what's being sent:
<SOAP-ENV:Envelope xmlns:SOAP-ENV="schemas.xmlsoap.org/soap/envelope/" xmlns:ns1="tempuri.org/"> <SOAP-ENV:Body> <ns1:AddSubscriber/> <LastName>MyLastName</LastName> <Email>[email protected]</Email> </SOAP-ENV:Body> </SOAP-ENV:Envelope>
I'm not very familar with SOAP, and I've been looking for documentation all over the place, but I can't seem to find a very good reference for what I'm doing.
Any guidance would be very much appreciated!
Thanks. Could you give me an example? I'm looking at the example on the PHP site that shows:
<?php class SOAPStruct { function SOAPStruct($s, $i, $f) { $this->varString = $s; $this->varInt = $i; $this->varFloat = $f; } } $client = new SoapClient(null, array('location' => "http://localhost/soap.php", 'uri' => "http://test-uri/")); $struct = new SOAPStruct('arg', 34, 325.325); $soapstruct = new SoapVar($struct, SOAP_ENC_OBJECT, "SOAPStruct", "http://soapinterop.org/xsd"); $client->echoStruct(new SoapParam($soapstruct, "inputStruct")); ?>
Are you saying I would have to create a Subscriber PHP class, assign all the vars
$this->FirstName = $first_name
, etc... and then put it in a SoapVar with encodingSOAP_ENC_OBJECT
? How can I better represent the subscriber structure?