PHP TCPDF Multiple WriteHTML() Not Working
Your problem is that after the $pdf->Output('exmpl/example_045.pdf', 'I')
, and as if the script ends there. Why shoot to the file header.
You can not shoot at two different header files, if you want to generate two distinct pdf I recommend you go with the function outputs the string
$pdf->Output('', 'S');
thus these two files on the filesystem save them and shoot. Compress the files and shoot the zipped file header containing the two pdf.
UPDATE
in your case I would use a similar approach:
function createPDF($html, $type, $filename, $output)
{
$pdf = new TCPDF();
//... code ...
if($type == "all"){
$pdf->writeHTML($html, true, false, false, false, '');
} elseif($type == "partial"){
$parts = explode("<div class='subTable'>", $html);
$pdf->writeHTML($parts[2], true, 0, true, 0);
}
$pdf->Output('exmpl/' . $filename . '.pdf', $output);
}
createPDF($html, "partial", "example_048", "F");
createPDF($html, "all", "example_046", "F");
createPDF($html, "all", "example_045", "I");
Final Update
right code:
<?php
//REQUIRE TCPDF
require_once('tcpdf/config/lang/eng.php');
require_once('tcpdf/config/lang/heb.php');
require_once('tcpdf/tcpdf.php');
class MYPDF extends TCPDF
{
//Page header
public function Header()
{
// Set font
$this->SetFont('helvetica', 'B', 20);
// Title
$this->Cell(0, 15, '<< TCPDF Example 003 >>', 0, false, 'C', 0, '', 0, false, 'M', 'M');
}
// Page footer
public function Footer()
{
// Position at 15 mm from bottom
$this->SetY(-15);
// Set font
$this->SetFont('helvetica', 'I', 8);
// Page number
$this->Cell(0, 10, 'Page ' . $this->getAliasNumPage() . '/' . $this->getAliasNbPages(), 0, false, 'C', 0, '', 0, false, 'T', 'M');
}
// Create PDF
function createPDF($html, $type, $filename, $output, $saleStart, $saleEnd, $reportType)
{
// create new PDF document
$pdf = new TCPDF(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false);
// set document information
/*
$pdf->SetCreator(PDF_CREATOR);
$pdf->SetAuthor('Nicola Asuni');
$pdf->SetTitle('TCPDF Example 018');
$pdf->SetSubject('TCPDF Tutorial');
$pdf->SetKeywords('TCPDF, PDF, example, test, guide');
*/
// SET CUSTOMIZED DATE INCLUDED HEADER
$headerSDate = reverseLongDate($saleStart);
$headerEDate = reverseLongDate($saleEnd);
$header = "";
$header .= "\t";
$header .= Date("d-m-Y h:m:s");
$header .= "\n";
$header .= 'מועדון המגדלים יוסי חותה פירות וירקות';
$header .= "\n";
if ($reportType == 'customer') {
$header .= 'דו"ח שיווק ללקוח לתאריכים ' . $headerSDate . " עד " . $headerEDate;
}
elseif ($reportType == 'newCustomer') {
$header .= 'דו"ח שיווק ללקוח לתאריכים ' . $headerSDate . " עד " . $headerEDate;
}
elseif ($reportType == 'collection') {
$header .= 'דו"ח גביה ללקוח לתאריכים ' . $headerSDate . " עד " . $headerEDate;
}
elseif ($reportType == 'grower') {
$header .= 'דו"ח מגדל ללקוח לתאריכים ' . $headerSDate . " עד " . $headerEDate;
}
$pdf->SetHeaderData("", "", "", $header);
//$pdf->SetHeaderData(PDF_HEADER_LOGO, PDF_HEADER_LOGO_WIDTH, PDF_HEADER_TITLE, PDF_HEADER_STRING);
// set header and footer fonts
$pdf->setHeaderFont(Array("dejavusans", '', "15"));
$pdf->setFooterFont(Array("dejavusans", '', "12"));
// set default monospaced font
$pdf->SetDefaultMonospacedFont(PDF_FONT_MONOSPACED);
//set margins
$pdf->SetMargins(PDF_MARGIN_LEFT, PDF_MARGIN_TOP, PDF_MARGIN_RIGHT);
$pdf->SetHeaderMargin(0);
$pdf->SetFooterMargin(PDF_MARGIN_FOOTER);
//set auto page breaks
$pdf->SetAutoPageBreak(TRUE, PDF_MARGIN_BOTTOM);
//set image scale factor
$pdf->setImageScale(PDF_IMAGE_SCALE_RATIO);
// set some language dependent data:
$lg = Array();
$lg['a_meta_charset'] = 'UTF-8';
$lg['a_meta_dir'] = 'rtl';
$lg['a_meta_language'] = 'fa';
$lg['w_page'] = 'page';
//set some language-dependent strings
$pdf->setLanguageArray($lg);
// ---------------------------------------------------------
// set font
$pdf->SetFont('dejavusans', '', 12);
// add a page
$pdf->AddPage();
// -----------------------------------------------------------------------------
if ($type == "all") {
$pdf->writeHTML($html, true, false, false, false, '');
} elseif ($type == "partial") {
$parts = explode("<div class='subTable'>", $html);
$pdf->writeHTML($parts[2], true, 0, true, 0);
}
ob_clean();
$pdf->Output('exmpl/' . $filename . '.pdf', $output);
} //END_OF_FUNCTION
}
set_time_limit(0);
require('modifyDate.php');
require('createQuery.php');
//GET FORM DATA
if (isset($_POST['submitMeshavek'])) {
$saleStart = $_POST['saleStart'];
$saleEnd = $_POST['saleEnd'];
$saleWeek = $_POST['weekId'];
$growerId = $_POST['growerId'];
$reportType = $_POST['reportType'];
}
if ($reportType == 'customer') {
include('reportType/customer.php');
}
elseif ($reportType == 'newCustomer') {
include('reportType/customerNew.php');
}
elseif ($reportType == 'collection') {
include('reportType/collect.php');
}
elseif ($reportType == 'grower') {
include('reportType/grower.php');
}
$myPdf = new MYPDF();
$myPdf->createPDF($html, "partial", "example_048", "F", $saleStart, $saleEnd, $reportType);
$myPdf->createPDF($html, "all", "example_046", "F", $saleStart, $saleEnd, $reportType);
$myPdf->createPDF($html, "all", "example_045", "I", $saleStart, $saleEnd, $reportType);
?>
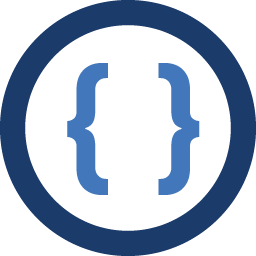
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I've written some code which created reports and then exports it to .PDF using TCPDF. I want to send different parts of the report to mail , therefor I need to use the function WriteHTML() more than once in order to create multiple pdf files and save them in a specific directory.
$parts=explode("<div class='subTable'>",$html); pdf->writeHTML($html, true, false, false, false, ''); $pdf->Output('exmpl/example_045.pdf', 'I'); $pdf->Output('exmpl/example_046.pdf', 'F'); $pdf->writeHTML($parts[2], true, 0, true, 0); //Close and output PDF document $pdf->Output('exmpl/example_048.pdf', 'F');
Altough I try to export two different files to the directory exmpl , all I actually get is one file (example_046.pdf).
I have to mention that I can use Output() more than once and I've already done such thing, but I just can't use writeHTML() more than once.
Any solution will be appreciated, Thanks in advance !
EDIT
Here's the full code :
<?php function createPDF($html, $type, $filename, $output,$saleStart,$saleEnd,$reportType) { //REQUIRE TCPDF require_once('tcpdf/config/lang/eng.php'); require_once('tcpdf/config/lang/heb.php'); require_once('tcpdf/tcpdf.php'); class MYPDF extends TCPDF { //Page header public function Header() { // Set font $this->SetFont('helvetica', 'B', 20); // Title $this->Cell(0, 15, '<< TCPDF Example 003 >>', 0, false, 'C', 0, '', 0, false, 'M', 'M'); } // Page footer public function Footer() { // Position at 15 mm from bottom $this->SetY(-15); // Set font $this->SetFont('helvetica', 'I', 8); // Page number $this->Cell(0, 10, 'Page '.$this->getAliasNumPage().'/'.$this->getAliasNbPages(), 0, false, 'C', 0, '', 0, false, 'T', 'M'); } } // create new PDF document $pdf = new TCPDF(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false); // set document information /* $pdf->SetCreator(PDF_CREATOR); $pdf->SetAuthor('Nicola Asuni'); $pdf->SetTitle('TCPDF Example 018'); $pdf->SetSubject('TCPDF Tutorial'); $pdf->SetKeywords('TCPDF, PDF, example, test, guide'); */ // SET CUSTOMIZED DATE INCLUDED HEADER $headerSDate=reverseLongDate($saleStart); $headerEDate=reverseLongDate($saleEnd); $header=""; $header.="\t"; $header.=Date("d-m-Y h:m:s"); $header.="\n"; $header.='מועדון המגדלים יוסי חותה פירות וירקות'; $header.="\n"; if($reportType=='customer') { $header.='דו"ח שיווק ללקוח לתאריכים '.$headerSDate." עד ".$headerEDate; } else if($reportType=='newCustomer') { $header.='דו"ח שיווק ללקוח לתאריכים '.$headerSDate." עד ".$headerEDate; } else if($reportType=='collection') { $header.='דו"ח גביה ללקוח לתאריכים '.$headerSDate." עד ".$headerEDate; } else if($reportType=='grower') { $header.='דו"ח מגדל ללקוח לתאריכים '.$headerSDate." עד ".$headerEDate; } $pdf->SetHeaderData("","" ,"",$header); //$pdf->SetHeaderData(PDF_HEADER_LOGO, PDF_HEADER_LOGO_WIDTH, PDF_HEADER_TITLE, PDF_HEADER_STRING); // set header and footer fonts $pdf->setHeaderFont(Array("dejavusans", '', "15")); $pdf->setFooterFont(Array("dejavusans", '', "12")); // set default monospaced font $pdf->SetDefaultMonospacedFont(PDF_FONT_MONOSPACED); //set margins $pdf->SetMargins(PDF_MARGIN_LEFT, PDF_MARGIN_TOP, PDF_MARGIN_RIGHT); $pdf->SetHeaderMargin(0); $pdf->SetFooterMargin(PDF_MARGIN_FOOTER); //set auto page breaks $pdf->SetAutoPageBreak(TRUE, PDF_MARGIN_BOTTOM); //set image scale factor $pdf->setImageScale(PDF_IMAGE_SCALE_RATIO); // set some language dependent data: $lg = Array(); $lg['a_meta_charset'] = 'UTF-8'; $lg['a_meta_dir'] = 'rtl'; $lg['a_meta_language'] = 'fa'; $lg['w_page'] = 'page'; //set some language-dependent strings $pdf->setLanguageArray($lg); // --------------------------------------------------------- // set font $pdf->SetFont('dejavusans', '', 12); // add a page $pdf->AddPage(); // ----------------------------------------------------------------------------- if($type == "all"){ $pdf->writeHTML($html, true, false, false, false, ''); } elseif($type == "partial"){ $parts = explode("<div class='subTable'>", $html); $pdf->writeHTML($parts[2], true, 0, true, 0); } ob_clean(); $pdf->Output('exmpl/' . $filename . '.pdf', $output); } //END_OF_FUNCTION set_time_limit(0); require('modifyDate.php'); require('createQuery.php'); //GET FORM DATA if(isset($_POST['submitMeshavek'])) { $saleStart=$_POST['saleStart']; $saleEnd=$_POST['saleEnd']; $saleWeek=$_POST['weekId']; $growerId=$_POST['growerId']; $reportType=$_POST['reportType']; } /* $regularSaleStart=reverseDate($saleStart); $regularSaleEnd=reverseDate($saleEnd); $header='<h2>מועדון מגדלים יוסי חותה פירות וירקות</h2>'; $header.="דוח שיווק ללקוח לתאריכים ".$regularSaleEnd." ".$regularSaleStart; public function Header() { // Set font $this->SetFont('dejavusans', '', 10); // Title $this->Cell(0, 15, '<< TCPDF Example 003 >>', 0, false, 'C', 0, '', 0, false, 'M', 'M'); } */ if($reportType=='customer') { include('reportType/customer.php'); } else if($reportType=='newCustomer') { include('reportType/customerNew.php'); } else if($reportType=='collection') { include('reportType/collect.php'); } else if($reportType=='grower') { include('reportType/grower.php'); } createPDF($html, "all", "example_045", "I",$saleStart,$saleEnd,$reportType); createPDF($html, "partial", "example_048", "F",$saleStart,$saleEnd,$reportType); createPDF($html, "all", "example_046", "F",$saleStart,$saleEnd,$reportType); /* ob_clean(); //$pdf->writeHTML($html, true, false, false, false, ''); $pdf->writeHTML($html, true, 0, true, 0); //Close and output PDF document $pdf->Output('example_048.pdf', 'I'); $pdf->writeHTML($html, true, 0, true, 0); //Close and output PDF document $pdf->Output('examp.pdf', 'I'); */ ?>