PHPUnit - 'No tests executed' when using configuration file
Solution 1
For what it's worth (being late), I ran into this recently while I was making a new Laravel 5.1 project for a simple website. I tried to debug it and was confused when I tried:
php artisan make:test homeTest
(which has a default test that just asserts true is true)
and saw the output
No tests executed!
What the problem ended up being for me was related to my PHP installation -- "phpunit" was globally registered and configured differently, whereas the phpunit that came with the Laravel installation was configured just right and ran perfectly.
So the fix is running the vendor's configured phpunit (from the same root directory as app/ and tests/):
./vendor/bin/phpunit
Hope that helps someone else!
Solution 2
Your XML file is fine as it is. However, you have to make sure that the PHP files in your tests/
folder are named as follows:
tests/Test.php <--- Note the uppercase "T"
tests/userTest.php
tests/fooBarTest.php
etc.
The filenames must end with "Test.php". This is what PHPUnit is looking for within directories.
Furthermore, every test method must either have a name that starts with "test" OR an @test
annotation:
public function testFooBar()
{
// Your test code
}
or:
/**
* @test
*/
public function fooBarTest() {
// test code here
}
Hope that helps!
Solution 3
On windows use the following command on terminal
.\vendor\bin\phpunit
that's if the command
phpunit
returns "No tests executed!"
while on Mac
./vendor/bin/phpunit
Hope it helps.
Solution 4
I had the same problem after PHPUnit on our virtual machines updated to version 6. Even --debug and --verbose said nothing useful, just "No tests executed". In the end it turned out that classes and namespaces were changed in the new version and it just didn't want to execute the files that contained references to old classes. The fix for me was just to replace in every test case this:
class MyTestCase extends \PHPUnit_Framework_TestCase {...}
with:
use PHPUnit\Framework\TestCase;
class MyTestCase extends TestCase {...}
Solution 5
I realize this is super old, but it just happened to me too. Hopefully this will help someone.
My problem was that I forgot the '@' symbol in /** @test */
WRONG:
/** test */
function a_thread_can_be_deleted()
{
...
}
RIGHT:
/** @test */
function a_thread_can_be_deleted()
{
...
}
Related videos on Youtube
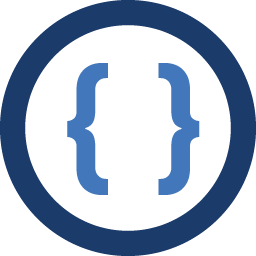
Muyiwa Olu
Updated on April 14, 2022Comments
-
Muyiwa Olu about 2 years
The Problem
To improve my quality of code, I've decided to try to learn how to test my code using Unit Testing instead of my mediocre-at-best testing solutions.
I decided to install PHPUnit using composer for a personal library that allows me to achieve common database functions. At first I didn't have a configuration file for PHPUnit and when I ran commands like:
$ phpunit tests/GeneralStringFunctions/GeneralStringFunctionsTest
Please note that this is a terminal command, so I didn't include the
.php
extension. The GeneralStringFunctionsTest referred to above is actually aGeneralStringFunctionsTest.php
file.The output is what I expected:
Time: 31 ms, Memory: 2.75Mb
OK (1 test, 1 assertion)
I then tried to use a configuration file to automatically load the test suite instead of having to manually type in the file every time. I created a file called
phpunit.xml
in my root directory, and entered the following into the file: http://pastebin.com/0j0L4WBD:<?xml version = "1.0" encoding="UTF-8" ?> <phpunit> <testsuites> <testsuite name="Tests"> <directory>tests</directory> </testsuite> </testsuites> </phpunit>
Now, when I run the command:
phpunit
I get the following output:
PHPUnit 4.5.0 by Sebastian Bergmann and contributors.
Configuration read from /Users/muyiwa/Projects/DatabaseHelper/phpunit.xml
Time: 16 ms, Memory: 1.50Mb
No tests executed!
In case it's helpful, my directory structure is as follows:
src - Top level directory (with all my source code)
tests - Top level directory (with all my tests, structured the same as my src folder)
vendor - Composer third party filesI also have the composer json and lock file, as well as the phpunit xml file in the top level as files.
Things I've Tried
- Changing the directory in
phpunit.xml
totests/GeneralStringFunctions
- Changing the directory in
phpunit.xml
to./tests
- Moving the
phpunit.xml
file to thetests
directory and then changing the directory to be./
instead oftests
. - Adding a suffix attribute to the directory tag in
phpunit.xml
to specify "Tests" as the explicit suffix.
-
hek2mgl about 9 yearsIs
tests/GeneralStringFunctions/GeneralStringFunctionsTest
a folder or a file name? -
Admin about 9 years@hek2mgl It's a filename, it's actually called
GeneralStringFunctionsTest.php
. In the command line interface, I didn't enter the.php
extension because it worked without it. -
hek2mgl about 9 yearsOK, then your configuration should work. Btw, if you specify a suffix it should be
Test.php
rather thanTest
in your case, but however, you are free to omit that sinceTest.php
is the default value. -
Admin about 9 years@hek2mgl Thanks for the heads up! Do you have any idea why my test isn't running with the configuration file
phpunit.xml
? -
ThW about 9 yearsWhy don't you show us the configuration file?
-
Admin about 9 years@ThW Hi, I did - sorry if it wasn't too clear: pastebin.com/0j0L4WBD.
-
hakre about 9 yearsWhich operating system are you using? which PHP version?
-
Admin about 9 years@hakre Hey, I am using PHP 5.6.2 and OS X Yosemite.
- Changing the directory in
-
Admin about 9 yearsHi, sorry if I didn't make it clear in the question, but I did add a test suite and corresponding name to the XML file. A copy can be found here: pastebin.com/0j0L4WBD.
-
Aine about 9 yearsTry <directory>./tests</directory>
-
Admin about 9 yearsThanks for the update, unfortunately I still get the same problem with ./tests as my directory.
Time: 28 ms, Memory: 1.50Mb No tests executed!
-
Admin about 9 yearsUnfortunately the same problem :(
Configuration read from /Users/muyiwa/Projects/Web Development/DatabaseHelper/phpunit.xml Time: 66 ms, Memory: 1.50Mb No tests executed!
. It definitely works when I manually reference it, it just doesn't like loading from the configuration file for some reason :( -
Aine about 9 yearsLast guess. Try moving the phpunit.xml file into the tests/ directory. In the file, change the directory to <directory>./</directory>.
-
Admin about 9 yearsStill the same output, I'm afraid. I can't get my head round it. Works perfectly when I manually call the file in a command argument, i.e.
phpunit tests/GeneralStringFunctions/GeneralStringFunctionsTest
. -
Steven Scott about 9 yearsTry using a complete path in the phpunit.xml file to your test directory. I believe the PHPUnit batch file on Windows changes the base directory you are running in, so your relative path ('./tests') may not be correct. If you are not on Windows, the relative path could still be the issue.
-
hakre about 9 years@Aine: There is no need to prefix the (already relative) path to the directory with "
./
". Just FYI. -
Admin about 9 years@StevenScott Something slightly different is happening now. I updated my
.bash_profile
to use my MAMP's PHP version, and I also made sure to update thephp.ini
file to remove short tags. Now when I run it, there are no tests, but it echoes out the content of the xml file like and then says no tests executed. -
Admin about 9 years
<?xml version="1.0" encoding="UTF-8" ?> <phpunit> <testsuites> <testsuite name="Tests"> <directory>/Users/muyiwa/Projects/webdev/DatabaseHelper/phpunit.xml</directory> </testsuite> </testsuites> </phpunit>PHPUnit 4.5.0 by Sebastian Bergmann and contributors. Configuration read from /Users/muyiwa/Projects/webdev/DatabaseHelper/phpunit.xml Time: 77 ms, Memory: 1.50Mb No tests executed!
-
checksum almost 8 yearsor you can use
--test-suffix=anything.php
to override -
Admin about 7 yearsI think this is most likely the correct answer. I've since removed the project so I have no way to double check, but for future projects with composer, I've always used
vendor/bin/phpunit
instead of the globalphpunit
binary. I've even gone as far as removing the globalphpunit
binary so I don't accidentally make the same mistake again -- and it hasn't popped up since. I shall mark this as the accepted answer, as it most likely is the reason. -
ira almost 7 yearscalling
./vendor/bin/phpunit
did the trick for me on a fresh laravel 5.4 install -
Tama almost 7 yearsAh, yes, while it doesn't look like this was the answer to the original question it solved my issue after upgrading to 6.x!
-
sqsinger almost 7 yearsSimilarly, this helped me while working on a Wordpress project. The same problem: "phpunit" can be globally registered and configured differently. Thank you for sharing!
-
Timur Samkharadze over 6 yearsfor me it was useful to replace relative path to test folder with absolute path.
-
Noitidart over 6 yearsThis fixed it for me, but is there a way to globally fix the phpunit? It would be nice if i can just type
phpunit
-
Ganesh K over 6 years@Noitidart you can create an
alias
for./vendor/bin/phpunit
-
kiwicomb123 over 6 yearsIs there a way to configure the global phpunit so that I can just call phpunit? (assuming I am not using composter and do not have the vender dir)
-
Wesley Brian Lachenal about 6 yearsThat alias thing works but when you have to use [--filter] or some sort. It just calls everything and it ignores that.
-
RoboBear about 6 years@kiwicomb123 - One idea is you could try updating your alias or command for running PHPUNIT and specfiy the config file in that command, such as "phpunit --configuration /path/to/config.xml". As for the "--filter" issue -- which I think is to specify one test or part of a test to run? -- you could try reading phpunit.de/manual/current/en/appendixes.configuration.html for help on using <filter>...</filter> blocks instead of on the command line. That way when you run via specifying the config file it should also parse the correct filter rules.
-
Goke Obasa about 6 yearsThanks so much for your answer, this really helps.
-
WindSaber over 5 yearsGreat, I was able to run test after that
-
Jonathan about 5 yearsHow do I override this in the configuration file?
-
aibarra over 4 yearsover 2 years later, and this was it for me. it really has to be exactly that format for the comment. i had a missing space in there. it didn't detect it. what's a little annoying, in laravel 6.1 i used the artisan make command and it doesn't include that comment
-
giovannipds over 4 yearsBe sure there's no
phpunit
at your global path (you can check it running something likephpunit --version
and see if it's matching to what're you expecting. Xampp usually installs a phpunit at the same php bin dir. That was the problem to me. -
giovannipds over 4 yearsOn windows you can check if there's no
phpunit
already running globally. Xampp usually puts a phpunit at the same php bin dir and that can be a problem. You can check phpunit version runningphpunit --version
. -
Liga over 4 yearsusing
vendor\bin\phpunit
(on Windows) worked for me! -
Sundar almost 4 yearsThis answer is useful
-
DAMIEN JIANG over 3 yearsI run into the problem when I was developing a package. When I run 'php artisan make:test SomeTest' and moved it to the package tests directory, the basic testExample method's comment only contains a basic description and
@return void
and doesn't contain '@test', which is the reason phpunit couldn't identify it as a test. -
confirmator over 3 yearsThis was the issue for me when upgrading from PHPUnit 5.7 to 8.5 as well - thank you for the tip!
-
JRod over 3 yearsI was trying to get it working with phpunit defined global and finally work in this way, I'm using MacOS and the command line is
phpunit --debug ./
-
Mycodingproject over 3 yearsI'm one of the most ignorant person in the world when it comes to unit testing so it would be nice the reason for this but it really helped. Thanks anyway!
-
Babak Asadzadeh about 3 yearsyou saved my day
-
Admin about 2 yearsAs it’s currently written, your answer is unclear. Please edit to add additional details that will help others understand how this addresses the question asked. You can find more information on how to write good answers in the help center.
-
clockw0rk about 2 yearswhy remembering suffix magic when you can just name the file AcceptanceXYZTest.php - this way everybody will instantly know that it's a test. You can ofc. be super unique and call it XYZTestCaseFile.php and use a suffix - but where's the net gain here?
-
Alkari almost 2 yearsAdding test to the methods fixed it, thank you