pip3 "TypeError: 'module' object is not callable" after update
Solution 1
From the link by Bram, I just ran python3 -m pip uninstall pip
, and it started to work again.
Solution 2
Use this
python -m pip install --upgrade --user [name_of_your_package]
Solution 3
The solution which worked for my situation is simply editing the pip3.8 file in the ubuntu environment.
Method1:
#!/path/to/.venv/bin/python3
# -*- coding: utf-8 -*-
import re
import sys
from pip._internal.main import main # <--- look at this import statement!
if __name__ == '__main__':
sys.argv[0] = re.sub(r'(-script\.pyw?|\.exe)?$', '', sys.argv[0])
sys.exit(main())
method2:
The main function has to be imported or we can simply replace line
sys.exit(main())
As
sys.exit(main.main())
Solution 4
As seen here, you should be able to solve this by running the module from Python directly, i.e.
python -m pip install --upgrade pip
Solution 5
In Windows edit C:\ProgramData\Anaconda3\Scripts\pip-script.py and replace
# -*- coding: utf-8 -*-
import re
import sys
from pip._internal import main
if __name__ == '__main__':
sys.argv[0] = re.sub(r'(-script\.pyw?|\.exe)?$', '', sys.argv[0])
sys.exit(main())
Replace the last line with sys.exit(main.main()).
Related videos on Youtube
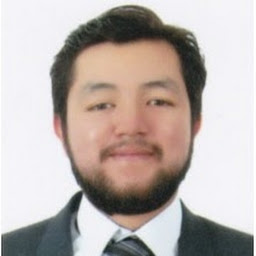
Israel Obando Cisneros
Updated on June 19, 2022Comments
-
Israel Obando Cisneros almost 2 years
I am new in Python, I wanna install Jupyter Notebook in my console I enter the following:
pip3 install --upgrade pip
after that I have a error to use pip3 install other library, the console print:
File "/usr/bin/pip3", line 11, in <module> sys.exit(main()) TypeError: 'module' object is not callable
I don't know what I have to do.
I use
sudo autoremove python3-pip
after that I usesudo apt install python3-pip
-
furas over 4 yearsmaybe it imports some of your file instead expected module and it has problem to run it - it can be ie.
main.py
. Did you try to usepip
in different folder ? -
Hyrial over 4 yearsI also had the same error.
-
jvonehr over 4 yearsThanks for getting me started - I listed my procedure for fixing this on stackoverflow.com/questions/34573159/…
-
-
Saif over 4 yearsCan somebody explain this?
-
vlz over 4 years@SaifUrRahman: From the provided link it seems that this is a conflict between a user specific pip installation (which gets installed/upgraded by the OPs command) and the global pip installation provided by the operating system.
-
Jetpack over 4 yearsThanks! method #2 worked for me. I'm nervous about it, because I'm afraid it will fail the next time I update /Applications/Xcode.app/Contents/Developer/usr/bin/pip3.
-
alpablo20 over 2 yearsfor some reason, I get a giant error starting with 'Could not install packages due to an EnvironmentError:" when I run
pip install pandas
for example. what could that be about? using the command @Hyrial suggested did help me install pandas with pip3, when before i got the same "module not callable" error.