Plot colored polygons with geodataframe in folium
Solution 1
I think I figured it out. In my previous code, polygon.get_facecolor() returns a list of RGBA values ranging from 0-1. I added this function (modified from this post):
def convert_to_hex(rgba_color) :
red = str(hex(int(rgba_color[0]*255)))[2:].capitalize()
green = str(hex(int(rgba_color[1]*255)))[2:].capitalize()
blue = str(hex(int(rgba_color[2]*255)))[2:].capitalize()
if blue=='0':
blue = '00'
if red=='0':
red = '00'
if green=='0':
green='00'
return '#'+ red + green + blue
to convert it to a hex string. Then:
gdf['RGBA'] = convert_to_hex(colors)
Then to plot the colors in folium, I do:
maploc = folium.Map(location=[42.377157,-71.236088],zoom_start=10,tiles="Stamen Toner")
colors = []
folium.GeoJson(
gdf,
style_function=lambda feature: {
'fillColor': feature['properties']['RGBA'],
'color' : feature['properties']['RGBA'],
'weight' : 1,
'fillOpacity' : 0.5,
}
).add_to(maploc)
and that created a really nice looking plot! (The property name is a bit misleading - it's not actually RGBA values, but hex strings.)
Solution 2
Not an expert... I just started with folium and jupyter and had a similar problem but with lines. You say you have GeoJson and polygons and the color is included in the json I assume.
The style_function might help you to get what you want?
The example below is produced with this page: http://geojson.io/ All I had to do was a "mapping" with the style_function. It's also possible to use a self defined function, see: https://github.com/python-visualization/folium/blob/master/examples/Colormaps.ipynb
import folium
geoJsonData = {
"features": [
{
"geometry": {
"coordinates": [
[
12.98583984375,
56.70450561416937
],
[
14.589843749999998,
57.604221411628735
],
[
13.590087890625,
58.15331598640629
],
[
11.953125,
57.955674494979526
],
[
11.810302734375,
58.76250326278713
]
],
"type": "LineString"
},
"properties": {
"stroke": "#fc1717",
"stroke-opacity": 1,
"stroke-width": 2
},
"type": "Feature"
},
{
"geometry": {
"coordinates": [
[
14.9468994140625,
57.7569377956732
],
[
15.078735351562498,
58.06916140721414
],
[
15.4302978515625,
58.09820267068277
],
[
15.281982421875002,
58.318144965188246
],
[
15.4852294921875,
58.36427519285588
]
],
"type": "LineString"
},
"properties": {
"stroke": "#1f1a95",
"stroke-opacity": 1,
"stroke-width": 2
},
"type": "Feature"
}
],
"type": "FeatureCollection"
}
m = folium.Map(location=[ 56.7, 12.9], zoom_start=6)
folium.GeoJson(geoJsonData,
style_function=lambda x: {
'color' : x['properties']['stroke'],
'weight' : x['properties']['stroke-width'],
'opacity': 0.6,
'fillColor' : x['properties']['fill'],
}).add_to(m)
m
The folium source code on git hub includes several nice examples as well:
https://github.com/python-visualization/folium/tree/master/examples
Here you find the options to play with:
http://leafletjs.com/reference.html#path-options
Hope this brings you forward!
Solution 3
I don't yet have enough reputation points to comment, so this is a separate answer to clarify the accepted answer written by edub.
Matplotlib has the colors.to_hex() method already:
import matplotlib.colors as cl
colors = [cl.to_hex(c) for c in colors]
This would replace the convert_to_hex() method in the accepted answer.
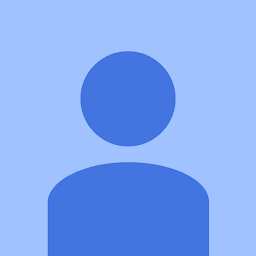
edub
Updated on July 09, 2022Comments
-
edub almost 2 years
I'm trying to plot radar data in folium, and I'm almost there. I followed this example (Contour plot data (lat,lon,value) within boundaries and export GeoJSON) to get my data into a GeoJson format.
nb_class = 20 collec_poly = plt.contourf(lons,lats,np.array(poshdata), nb_class,alpha=0.5) gdf = collec_to_gdf(collec_poly) # From link above gdf.to_json() colors = [p.get_facecolor().tolist()[0] for p in collec_poly.collections] gdf['RGBA'] = colors gdf
This outputs two columns: geometry and RGBA.
RGBA geometry 0 [0.0, 0.0, 0.713903743316, 1.0] (POLYGON ((-71.57032079644679 42.2775236331535... 1 [0.0, 0.0960784313725, 1.0, 1.0] (POLYGON ((-71.56719970703125 42.2721176147460... 2 [0.0, 0.503921568627, 1.0, 1.0] (POLYGON ((-71.55678558349609 42.2721176147460... 3 [0.0, 0.896078431373, 0.970904490829, 1.0] (POLYGON ((-71.52552795410156 42.2849182620049... 4 [0.325743200506, 1.0, 0.641998734978, 1.0] (POLYGON ((-71.49427795410156 42.2939676156927... 5 [0.641998734978, 1.0, 0.325743200506, 1.0] (POLYGON ((-71.47344207763672 42.3003084448852... 6 [0.970904490829, 0.959331880901, 0.0, 1.0] (POLYGON ((-71.26508331298828 42.3200411822557... 7 [1.0, 0.581699346405, 0.0, 1.0] (POLYGON ((-71.15048217773438 42.3333218460720...
From there I make my folium map:
import folium # Picked location between Sudbury and Somerville: maploc = folium.Map(location=[42.377157,-71.236088],zoom_start=11,tiles="Stamen Toner") folium.GeoJson(gdf).add_to(maploc)
This creates my nice folium map, but the polygons are not colored at all. How do I get the contours to be filled with the right colors? And fix the opacity?
-
Hoppeduppeanut almost 4 yearsNote that while you might not have enough reputation to comment yet, you can still edit other users' posts to include clarification, if necessary.
-
lead almost 4 yearsI know you aren't the one who sets this policy, but it seems out of line for me to edit somebody else's answer while I'm still unable to comment on their answer. I don't understand why this is the order of things.
-
Hoppeduppeanut almost 4 yearsI know, it's a bit silly, but it's to prevent extraneous or conversational comments (like adding "+1" or "thanks!!!" comments) and spam, as well as edits by newer users being peer reviewed by the community before they're made public, so there's a layer of protection there that comments don't have. You can discuss this with the wider community on Meta Stack Overflow once you earn 5 reputation, which you can do with a couple of approved edits or an upvoted question/answer.
-
lead almost 4 yearsI mean, I've been using StackOverflow for well over 2 years now, multiple times a day. I have 10s of reputation points in all the other communities I participate in far less frequently. It says a lot that I don't yet have the 5 reputation points required to comment on how one gets reputation points on this particular site.