Plot GeoIP data on a World Map
Solution 1
Perhaps Basemap in Matplotlib for the plotting.
It can do all sorts of projections (don't mind the ugly default colours). And you have good control over what/how you plot. You can even use NASA Blue Marble imagery. And of course, you can plot markers, lines, etc on the map using the plot command.
I haven't put huge datasets through it, but being that it is a part of Matplotlib I suspect it will do well.
If I remember right, despite being part of the library, by default it doesn't ship with Matplotlib.
Solution 2
As others have mentioned, you can fetch the locations and write some code to put them on a map, but there's also an easier way to do this.
You can use IPInfo's free Map IPs tool, which will create an interactive map of IP addresses. Here's an example for AWS IP Ranges.
You can either copy/paste the IPs or use the tool via cURL. It will process up to 500K IPs.
$ cat ipList | curl -XPOST --data-binary @- "ipinfo.io/map?cli=1"
Disclaimer: I work at IPInfo.
Solution 3
The google maps API can support all of the above if you would be willing to set up a local web environment for testing. There is a wrapper around the API for Python called pymaps.
The API documentation has an example on how to use Google's geocoder to plot points on a map given a particular input address:
Google Maps API Example: Simple Geocoding
Solution 4
You might want to have a look at mapsplotlib
.
Installation
ONLY PYTHON 2.7!
$ pip install mapsplotlib
$ pip install pandas
You'll then need to have a Google Static Maps API key, go to https://console.developers.google.com
Usage
import pandas as pd
from mapsplotlib import mapsplot as mplt
df = pd.read_csv("data.csv")
mplt.register_api_key('your_google_api_key_here')
mplt.density_plot(df['latitude'], df['longitude'])
Example output
Solution 5
I recently used gmplot
.
Simply install it with
pip install gmplot
It is extremely easy to use. All you have to do is plug in the longitude and latitude values and gmplot
will put out a map in html-form.
Here's a minimal example. Let lat_values
and lon_values
be lists of latitude and longitude values.
from gmplot import gmplot
import numpy as np
center_lat = np.mean(lat_values)
center_lon = np.mean(lon_values)
zoom = 15
gmap = gmplot.GoogleMapPlotter(center_lat, center_lon, zoom)
gmap.scatter(lat_values, lon_values)
gmap.draw('my_map.html')
That's it! Of course, you can tune parameters to enhance the visuals. See the crash course here.
Related videos on Youtube
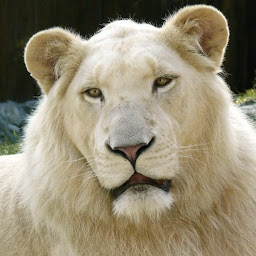
Awais Mahmood
Updated on April 21, 2022Comments
-
Awais Mahmood about 2 years
I need a library (preferably written in Python) which is able to take a series of IP addresses (or geographic coordinates) and plots them on a World Map.
I already found this one, but it outputs data as a .svg file and it's simply unable to cope with large data sets.
Please note that I need something which can be downloaded and run locally, so no data uploading to 3rd party web services.
Thanks!
-
Awais Mahmood over 14 yearsFrom what I understand, pymaps is just a wrapper to make using Google Maps easier. However, I want to run this completely offline, without sending any data outside (to Google for instance).
-
Kazuya Gosho almost 3 yearsipinfo.io is exactly what I was looking for. Thanks for sharing!!