Plotting graph using python and dispaying it using HTML
Solution 1
My suggestion would be to use the plotly.offline
module, which creates an offline version of a plot for you. The plotly API on their website is horrendous (we wouldn't actually want to know what arguments each function takes, would we??), so much better to turn to the source code on Github.
If you have a look at the plotly source code, you can see that the offline.plot
function takes a kwarg for output_type
, which is either 'file'
or 'div'
:
https://github.com/plotly/plotly.py/blob/master/plotly/offline/offline.py
So you could do:
from plotly.offline import plot
from plotly.graph_objs import Scatter
my_plot_div = plot([Scatter(x=[1, 2, 3], y=[3, 1, 6])], output_type='div')
This will give you the code (wrapped in <div>
tags) to insert straight into your HTML. Maybe not the most efficient solution (as I'm pretty sure it embeds the relevant d3 code as well, which could just be cached for repeated requests), but it is self contained.
To insert your div into your html code using Flask, there are a few things you have to do.
In your html template file for your results page, create a placeholder for your plot code. Flask uses the Jinja template engine, so this would look like:
<body>
....some html...
{{ div_placeholder }}
...more html...
</body>
In your Flask views.py
file, you need to render the template with the plot code inserted into the div_placeholder
variable:
from plotly.offline import plot
from plotly.graph_objs import Scatter
from flask import Markup
...other imports....
@app.route('/results', methods=['GET', 'POST'])
def results():
error = None
if request.method == 'POST':
my_plot_div = plot([Scatter(x=[1, 2, 3], y=[3, 1, 6])], output_type='div')
return render_template('results.html',
div_placeholder=Markup(my_plot_div)
)
# If user tries to get to page directly, redirect to submission page
elif request.method == "GET":
return redirect(url_for('submission', error=error))
Obviously YMMV, but that should illustrate the basic principle. Note that you will probably be getting a user request using POST data that you will need to process to create the plotly graph.
Solution 2
You can use the .to_html()
method:
import plotly.express as px
fig = px.scatter(x=[0, 1, 2, 3, 4], y=[0, 1, 4, 9, 16])
div = fig.to_html(full_html=False) # Get the <div> to send to your frontend and embed in an html page
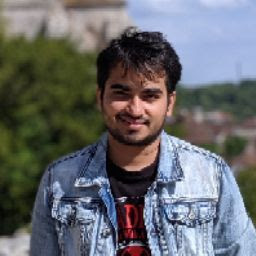
danish sodhi
Updated on July 14, 2022Comments
-
danish sodhi almost 2 years
I want build an offline application using plotly for displaying graphs . I am using python(flask) at the back end and HTML(javascript) for the front end . Currently I am able to plot the graph by sending the graph data as JSON object to front end and building the graph using plotly.js at the front end itself . But what I actually want is to build the graph at the server(backend ie python) side itself and then display the data in HTML . I have gone through the plotly documentation that builds the graph in python , but I dont know how to send the build graph to front end for display :( Can someone help me on that ? PS : I want to build an offline application Updated Code
$(window).resize(function() { var divheight = $("#section").height(); var divwidth = $("#section").width(); var update = { width:divwidth, // or any new width height:divheight // " " }; var arr = $('#section > div').get(); alert(arr[1]); Plotly.relayout(arr[0], update); }).resize(); });
-
danish sodhi almost 8 yearsHi Andrew , Thanks for answering :) Assuming that my_plot_div is a <div>, Can you guide me on how will I be able to transfer this div information to front end ? Earlier I used to transfer JSON(containig info like data , layout but still not plotted infromation) from server to front end for display ....How can I do in this case :( Thanks alot in advance :)
-
Andrew Guy almost 8 yearsI've edited my answer to include info on rendering this data with Flask.
-
danish sodhi almost 8 yearsThanks alot Andrew .... it was help full alot :) Cas I define a CSS for this div generated by the plotly .........Actually I want to resize my graph as my window resizes :)
-
danish sodhi almost 8 yearsActually can I fix the div Id of the plotly plot ?
-
Andrew Guy almost 8 yearsWhy not just do
<div id='plot_id'> {{ div_placeholder }} </div>
? -
danish sodhi almost 8 yearsI am doing like this only .... but I dont know how to resize graph like this ................... when I was using plotly.js(ie when I was not plotting in python ) I used to use the above updated code for resize ... Dont know how to do now :(
-
Andrew Guy almost 8 yearsI'm not sure about the answer to that. I haven't used
plotly
much at all, so might be worth posting another question with your specific requirements for resizing. -
danish sodhi almost 8 yearsThanks for your help .......one last question .... If I go with the above code ..... Are you sure that the plotting is happening in python or the data is being transferred to front end using jinja templtes and plotting is being done there using plotly.js(or smthing) at the front end ?
-
Andrew Guy almost 8 yearsThe creation of the javascript code (which uses the D3 library) to display your plot is being done in Python. The final javascript code needed to render the plot is then being passed to the front end. In this sense, the plotting is happening in JS. But unless you want to just render an image, there's no other way around it. Any heavy computational stuff will be being done in Python on the server side though, which I think is what you are aiming for.
-
A.P. over 5 yearsWhat is
Markup
? @AndrewGuy How do I import it? -
Andrew Guy over 5 years@A.P. I'm not sure what you are talking about. I can't see any reference to
Markup
in my answer. Can you clarify? -
A.P. over 5 years@AndrewGuy Really? I see this piece of code
return render_template('results.html', div_placeholder=Markup(my_plot_div) )
. I think I found itfrom flask import Markup
. Thanks still for taking time to come back to an old answer. -
Andrew Guy over 5 years@A.P. Sorry, missed that somehow.
Markup
is from the python Flask library.from flask import Markup
. I've edited the answer to include this. Thanks for highlighting this. -
Pirate X about 4 years@AndrewGuy Updated github link Plot.ly Offline