Populate 2nd dropdown based on selection from 1st dropdown in React.Js
You're doing it wrong way. You cannot call onClick method on <option>
. Instead you should use onChange method on <select>
(see react docs) like this:
<select onChange={this.handleChange} >
<option>1</option>
<option>2</option>
<option>3</option>
</select>
Then on 'onChange' event you can set your state to the selected option. Let's understand this scenario with an example.
Suppose you have two dropdowns. One for showing companies and one for jobs corresponding to each company. Each company has its own set of jobs like this:
companies:[
{ name: 'company1', jobs: ['job1-1', 'job1-2', 'job1-3']},
{ name: 'company2', jobs: ['job2-1', 'job2-2', 'job2-3']},
{ name: 'company3', jobs: ['job3-1', 'job3-2', 'job3-3']}
]
Now you have to just set a state, lets say 'selectedCompany' and then filter the companies array by 'selectedCompany'. Then you can just get that particular company object and map your 'jobs' dropdown by iterating over jobs array inside the company object.
Here is the code snippet: https://codepen.io/madhurgarg71/pen/pRpoMw
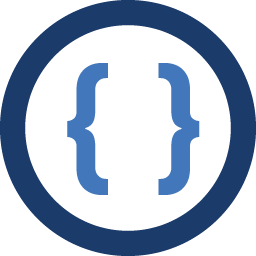
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I am learning react and struggling to get a second dropdown to populate based on which option is clicked from first dropdown.
I have included my code below.
I think the issue is where I try to set
this.state.selected = param.tableName
. I don't think that will work, but I'm not sure what to use instead.I need an
onClick
event to change thethis.state.selected
variable to the option that is selected I think, and then check whentableName === selected
. I will also include my JSON below so the context is more clear.import React from 'react'; import axios from 'axios'; class Search extends React.Component { constructor(props) { super(props) this.state = { params: [], columnParams: [], selected: '' } } componentWillMount() { axios.get('http://localhost:3010/api/schema') .then( response => { this.setState({params: response.data}) }) } render() { return ( <div className="Search"> <select>{this.state.params.map((param, i) => <option key={i} onClick={this.setState(this.state.selected ={param.tableName})}> {param.tableName} </option>)} </select> <select> { this.state.params .filter(({tableName}) => tableName === selected) .map(({columns}) => columns.map((col) =><option>{col}</option>))} [ { "tableName": "customer", "columns": [ "customerid", "title", "prefix", "firstname", "lastname", "suffix", "phone", "email" ] }, { "tableName": "product", "columns": [ "productid", "name", "color", "price", "productadjective", "productmaterial" ] },