Populate a form after submit with jQuery and ajax from
AJAX
Put this code between <head>
and </head>
tags of your form page and remember to call jquery framework.
<script type="text/javascript">
$( "#number" ).keyup(function() {
numberval = $('#number').val();
$.ajax({
type: "GET",
dataType: "json",
url: "PHP-FILE.php", // replace 'PHP-FILE.php with your php file
data: {number: numberval},
success: function(data) {
$('#name').val(data["name"]);
$("#email").val(data["email"]);
},
error : function(){
alert('Some error occurred!');
}
});
});
</script>
FORM
<form method="post" action="#">
<input name="number" type="text" id="number" value="" />
<input name="name" type="text" id="name" value="" />
<input name="email" type="text" id="email" value="" />
<button type"submit">
</form>
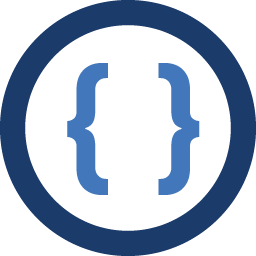
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I have a basic HTML form and want to preform a lookup with Ajax via a PHP file after filling in the first field, looking in an external feed for the values of the two second fields.
<form method="post" action="#"> <input name="number" type="text" id="number" value="" /> <input name="name" type="text" id="name" value="" /> <input name="email" type="text" id="email" value="" /> <button type"submit"> </form>
After filling in the first field (number), I want to do an Ajax call to a PHP file (submitting the filled in value) and look for the values of the two other fields (name and email). After the lookup fill in the found values in this form, user can edit if wanted and then submit.
The PHP file looks like this:
<?php $number = $_GET["number"]; $url = "http://api.domain.com/lookup/$number"; $response = json_decode(file_get_contents($url), true); ?>
And will give a json response like this
{ "name": "jacob", "email": "[email protected]" }
Now I need jQuery to complete this task (call the PHP script and populate the other form fields) and that's where I got stuck. Ideas?
-
Julo0sS over 8 yearsajaxCall is called only when focusout here... quick solution but not 100% effective... (Fill 2nd & 3rd fields 1st, then number and do not get out but just post form with enter & see)
-
Admin over 8 yearsIt will work for now, maybe it's better to add an extra button as well to retrieve the data in stead of onchange. Thanks for the help.
-
Maverick over 8 years@Dennis I added a keyup() binding so you don't need any extra button and all works well. ;)
-
Sherali Turdiyev over 8 yearsthere is
var obj = jQuery.parseJSON(data);
unnecessary