Populate listview using SimpleCursorAdapter in Android
Solution 1
The error you are getting is most likely because you're trying to embed a TextView into a ListView...
<ListView
android:layout_weight="1"
android:layout_height="wrap_content"
android:id="@+id/answerList"
android:layout_width="match_parent">
<TextView
android:id="@+id/answerItem"
android:layout_height="match_parent"
android:layout_width="match_parent">
</TextView>
</ListView>
Remove the TextView from the ListView and then try the following...
String[] from = new String[] {"answer"};
int[] to = new int[] {android.R.id.text1};
SimpleCursorAdapter cursorAdapter = new SimpleCursorAdapter(context, android.R.layout.simple_list_item_1, answers, from, to);
This uses the pre-defined Android android.R.layout.simple_list_item_1
and android.R.id.text1
Solution 2
Your to and from need to be the same length. You don't need to include _id in the 'from' array. Note that you still need to have _id in your cursor.
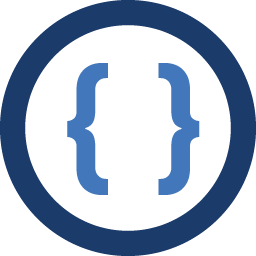
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I know this question has been asked and answered before, but I have followed multiple tutorials to no avail.
I just thought if I put my code up maybe someone could help and point me in the right direction. I am not going to lie, I am a massive noob at this but am loving it so far (well, up to this point anyway). All that is happening is when I load the activity, it just force closes with the following error message:
06-14 20:04:08.162: ERROR/AndroidRuntime(17605): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.magic8/com.example.magic8.AnswerList}: java.lang.UnsupportedOperationException: addView(View, LayoutParams) is not supported in AdapterView
So, here it is. My database query is:
public Cursor fetchAnswersList() { return mDb.query(DATABASE_TABLE, new String[] {KEY_ROWID, "answer"}, null, null, null, null, null, null); }
Then my list view code:
public class AnswerList extends Activity { private Context context; private DatabaseManager mDbHelper; public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.answerlist); Cursor answers = mDbHelper.fetchAnswersList(); startManagingCursor(answers); ListView answerList=(ListView)findViewById(R.id.answerList); String[] from = new String[] {"_id", "answer"}; int[] to = new int[] {R.id.answerItem}; SimpleCursorAdapter cursorAdapter = new SimpleCursorAdapter(context, R.layout.list_item, answers, from, to); answerList.setAdapter(cursorAdapter); answerList.setOnItemClickListener( new OnItemClickListener() { public void onItemClick(AdapterView<?> parent, View view, int position, long id) { // When clicked, show a toast with the TextView text setToast("Answer: " + ((TextView) view).getText()); } }); }
And last but not least, here is my XML:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical"> <LinearLayout android:id="@+id/addAnswerForm" android:layout_height="wrap_content" android:layout_width="match_parent" android:orientation="horizontal"> <EditText android:id="@+id/addAnswer" android:layout_weight="1" android:layout_height="wrap_content" android:layout_width="wrap_content"> <requestFocus></requestFocus> </EditText> <Button android:text="Add" android:id="@+id/addAnswer_btn" android:layout_width="wrap_content" android:layout_height="wrap_content"> </Button> </LinearLayout> <LinearLayout android:id="@+id/answers" android:layout_height="match_parent" android:layout_width="match_parent"> <ListView android:layout_weight="1" android:layout_height="wrap_content" android:id="@+id/answerList" android:layout_width="match_parent"> <TextView android:id="@+id/answerItem" android:layout_height="match_parent" android:layout_width="match_parent"> </TextView> </ListView> </LinearLayout> </LinearLayout>