PowerShell - Finding all of users' group memberships and kicking it out of them
Assuming that all backlinks are in place, this is a simple 3-step process easily done with powershell:
# 1. Retrieve the user in question:
$User = Get-ADUser "username" -Properties memberOf
# 2. Retrieve groups that the user is a member of
$Groups = $User.memberOf |ForEach-Object {
Get-ADGroup $_
}
# 3. Go through the groups and remove the user
$Groups |ForEach-Object { Remove-ADGroupMember -Identity $_ -Members $User }
If you don't want to manually confirm removing the user for each group, use -Confirm:$false
:
Remove-ADGroupMember -Identity $_ -Members $User -Confirm:$false
Might I add that you probably want to log every group membership you remove, just for the sake of easy recovery. Before removal, print the group DN's to a text file, identifying the user in question:
$LogFilePath = "C:\BackupLocation\user_" + $User.ObjectGUID.ToString() + ".txt"
Out-File $LogFilePath -InputObject $(User.memberOf) -Encoding utf8
This will write all the groups into the file and allow for easy and reliable rollback
Related videos on Youtube
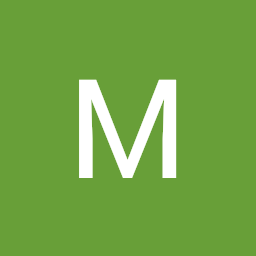
Mark
Updated on September 18, 2022Comments
-
Mark almost 2 years
I have this game environment made by third party. It has a play button such that every time I click on it certain things happen and a bunch of json data is generated. Right now I am trying to collect this data but I don't want to have to keep clicking the play button. I looked up How to auto click an input button, but I believe the solutions provided there all work only at the start of loading the window. My problem is that I only have the ability to modify the javascript from the
chrome developer tools
and I don't believe that the changes that are made in thesource's tab
persists once I refresh the page (I could be wrong but based on what I have observed so far that's what's happening). How can I get the code below to run multiple times (say just 10 for now) without clicking theStart Game
button while only modifying the code in the chrome developer tools?console.log("loading...") $(() => { $("#start-game-btn").click(event => { console.log("start-game") $("#errors").text("") event.preventDefault() const height = parseInt($("#height").val()) const width = parseInt($("#width").val()) const food = parseInt($("#food").val()) let MaxTurnsToNextFoodSpawn = 0 if ($("#food-spawn-chance").val()) { MaxTurnsToNextFoodSpawn = parseInt($("#food-spawn-chance").val()) } const snakes = [] $(".snake-group").each(function() { const url = $(".snake-url", $(this)).val() if (!url) { return } snakes.push({ name: $(".snake-name", $(this)).val(), url }) }) if (snakes.length === 0) { $("#errors").text("No snakes available") } fetch("http://localhost:3005/games", { method: "POST", body: JSON.stringify({ width, height, food, MaxTurnsToNextFoodSpawn, "snakes": snakes, }) }).then(resp => resp.json()) .then(json => { const id = json.ID fetch(`http://localhost:3005/games/${id}/start`, { method: "POST" }).then(_ => { $("#board").attr("src", `http://localhost:3009?engine=http://localhost:3005&game=${id}`) }).catch(err => $("#errors").text(err)) }) .catch(err => $("#errors").text(err)) }) console.log("ready!") })
I tried doing a while loop at the start like,
$(() => { var count = 0 while(count <= 10) { count++ console.log("start-game") $("#errors").text("") event.preventDefault() .........
but nothing changed.
Also I have no experience with jQuery and bare minimum knowledge on javascript so do bear with me. Thanks in advance.
-
Tim Ferrill about 10 yearsSo you're starting with a user, and wanting to remove its membership in all groups?
-
-
Npv23g about 10 yearsthe last operation (Go through the groups and remove the user) waits for more parameters (gives a
>>
output..) why?