Practical example of Polymorphism
Solution 1
Check the Wikipedia example: it is very helpful at a high level:
class Animal:
def __init__(self, name): # Constructor of the class
self.name = name
def talk(self): # Abstract method, defined by convention only
raise NotImplementedError("Subclass must implement abstract method")
class Cat(Animal):
def talk(self):
return 'Meow!'
class Dog(Animal):
def talk(self):
return 'Woof! Woof!'
animals = [Cat('Missy'),
Cat('Mr. Mistoffelees'),
Dog('Lassie')]
for animal in animals:
print animal.name + ': ' + animal.talk()
# prints the following:
#
# Missy: Meow!
# Mr. Mistoffelees: Meow!
# Lassie: Woof! Woof!
Notice the following: all animals "talk", but they talk differently. The "talk" behaviour is thus polymorphic in the sense that it is realized differently depending on the animal. So, the abstract "animal" concept does not actually "talk", but specific animals (like dogs and cats) have a concrete implementation of the action "talk".
Similarly, the "add" operation is defined in many mathematical entities, but in particular cases you "add" according to specific rules: 1+1 = 2, but (1+2i)+(2-9i)=(3-7i).
Polymorphic behaviour allows you to specify common methods in an "abstract" level, and implement them in particular instances.
For your example:
class Person(object):
def pay_bill(self):
raise NotImplementedError
class Millionare(Person):
def pay_bill(self):
print "Here you go! Keep the change!"
class GradStudent(Person):
def pay_bill(self):
print "Can I owe you ten bucks or do the dishes?"
You see, millionares and grad students are both persons. But when it comes to paying a bill, their specific "pay the bill" action is different.
Solution 2
A common real example in Python is file-like objects. Besides actual files, several other types, including StringIO and BytesIO, are file-like. A method that acts as files can also act on them because they support the required methods (e.g. read
, write
).
Solution 3
A C++ example of polymorphism from the above answer would be:
class Animal {
public:
Animal(const std::string& name) : name_(name) {}
virtual ~Animal() {}
virtual std::string talk() = 0;
std::string name_;
};
class Dog : public Animal {
public:
virtual std::string talk() { return "woof!"; }
};
class Cat : public Animal {
public:
virtual std::string talk() { return "meow!"; }
};
void main() {
Cat c("Miffy");
Dog d("Spot");
// This shows typical inheritance and basic polymorphism, as the objects are typed by definition and cannot change types at runtime.
printf("%s says %s\n", c.name_.c_str(), c.talk().c_str());
printf("%s says %s\n", d.name_.c_str(), d.talk().c_str());
Animal* c2 = new Cat("Miffy"); // polymorph this animal pointer into a cat!
Animal* d2 = new Dog("Spot"); // or a dog!
// This shows full polymorphism as the types are only known at runtime,
// and the execution of the "talk" function has to be determined by
// the runtime type, not by the type definition, and can actually change
// depending on runtime factors (user choice, for example).
printf("%s says %s\n", c2->name_.c_str(), c2->talk().c_str());
printf("%s says %s\n", d2->name_.c_str(), d2->talk().c_str());
// This will not compile as Animal cannot be instanced with an undefined function
Animal c;
Animal* c = new Animal("amby");
// This is fine, however
Animal* a; // hasn't been polymorphed yet, so okay.
}
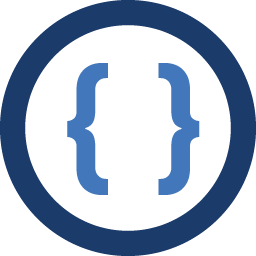
Admin
Updated on November 02, 2020Comments
-
Admin over 3 years
Can anyone please give me a real life, practical example of Polymorphism? My professor tells me the same old story I have heard always about the
+
operator.a+b = c
and2+2 = 4
, so this is polymorphism. I really can't associate myself with such a definition, since I have read and re-read this in many books.What I need is a real world example with code, something that I can truly associate with.
For example, here is a small example, just in case you want to extend it.
>>> class Person(object): def __init__(self, name): self.name = name >>> class Student(Person): def __init__(self, name, age): super(Student, self).__init__(name) self.age = age