pragma omp parallel for vs. pragma omp parallel
11,115
#pragma omp parallel
for(int i=0; i<N; i++) {
...
}
This code creates a parallel region, and each individual thread executes what is in your loop. In other words, you do the complete loop N times, instead of N threads splitting up the loop and completing all iterations just once.
You can do:
#pragma omp parallel
{
#pragma omp for
for( int i=0; i < N; ++i )
{
}
#pragma omp for
for( int i=0; i < N; ++i )
{
}
}
This will create one parallel region (aka one fork/join, which is expensive and therefore you don't want to do it for every loop) and run multiple loops in parallel within that region. Just make sure if you already have a parallel region you use #pragma omp for
as opposed to #pragma omp parrallel for
as the latter will mean that each of your N threads spawns N more threads to do the loop.
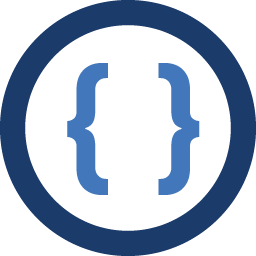
Author by
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
In C++ with openMP, is there any difference between
#pragma omp parallel for for(int i=0; i<N; i++) { ... }
and
#pragma omp parallel for(int i=0; i<N; i++) { ... }
?
Thanks!
-
Admin about 8 yearsI see; that's what I needed. So the first option (
#pragma omp parallel
) would execute Nthreads * N iterations in total, and any statement of the formvec[i] = result;
inside the loop would mess everything up. -
RyanP about 8 years@FranciscoJ.R.Ruiz that's correct. If this answer's your question, feel free to mark it as the answer :)
-
Admin about 8 yearsSure! Thanks a lot.