preparing Json Object for HttpClient Post method
12,525
Solution 1
You need to either manually serialize the object first using JsonConvert.SerializeObject
var values = new Dictionary<string, string>
{
{"type", "a"}, {"card", "2"}
};
var json = JsonConvert.SerializeObject(values);
var data = new StringContent(json, Encoding.UTF8, "application/json");
//...code removed for brevity
Or depending on your platform, use the PostAsJsonAsync
extension method on HttpClient
.
var values = new Dictionary<string, string>
{
{"type", "a"}, {"card", "2"}
};
var client = new HttpClient();
using(var response = client.PostAsJsonAsync(myUrl, values).Result) {
result = response.Content.ReadAsStringAsync().Result;
}
Solution 2
https://www.newtonsoft.com/json use this. there are already a lot of similar topics. Send JSON via POST in C# and Receive the JSON returned?
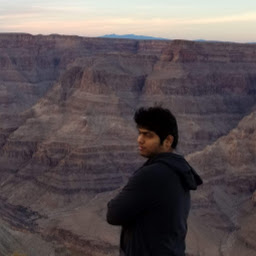
Author by
Rk R Bairi
Runs things using thoughts. Interested in tech and agriculture.
Updated on June 04, 2022Comments
-
Rk R Bairi about 2 years
I am trying to prepare a JSON payload to a Post method. The server fails unable to parse my data.
ToString()
method on my values would not convert it to JSON correctly, can you please suggest a correct way of doing this.var values = new Dictionary<string, string> { { "type", "a" } , { "card", "2" } }; var data = new StringContent(values.ToSttring(), Encoding.UTF8, "application/json"); HttpClient client = new HttpClient(); var response = client.PostAsync(myUrl, data).Result; using (HttpContent content = response.content) { result = response.content.ReadAsStringAsync().Result; }