Preparing simple multipart/alternative email using MimeMessageHelper (Spring Framework)
Solution 1
Because of a spam checker issue, I was looking to send html email with alternative but it seems that spring just don't provide a simple MimeMessageHelper.MULTIPART_MODE_ALTERNATIVE. However using the MimeMessageHelper.MULTIPART_MODE_MIXED_RELATED get my emails reach their destinations.
If you still want to get rid of mixed and related boundaries, you need more control over how a MIME message is assembled, you can create an implementation of the MimeMessagePreparator interface and pass this to the JavaMailSender.send() method in place of the MimeMessage.
sender.send(new MessagePreparator());
private class MessagePreparator implements MimeMessagePreparator {
public void prepare(MimeMessage msg) throws Exception {
// set header details
msg.addFrom(InternetAddress.parse(from));
msg.addRecipients(Message.RecipientType.TO, InternetAddress.parse(to));
msg.setSubject(subject);
// create wrapper multipart/alternative part
MimeMultipart ma = new MimeMultipart("alternative");
msg.setContent(ma);
// create the plain text
BodyPart plainText = new MimeBodyPart();
plainText.setText("This is the plain text version of the mail.");
ma.addBodyPart(plainText);
// create the html part
BodyPart html = new MimeBodyPart();
html.setContent(
"<html><head></head><body>
<h1>This is the HTML version of the mail."
+ "</h1></body></html>", "text/html");
ma.addBodyPart(html);
}
}
}
The order in which you add BodyPart instances to the MimeMultipart is important, and you should add the BodyPart with the most preferable message format last.
This is an excerpt taken from Pro Spring 2.5 chapter13 - § Sending an HTML Message with a Plain Text Alternative ; APRESS ISBN-13 (pbk): 978-1-59059-921-1
Solution 2
There is also simper way to do that:
MimeMessage message = javaMailSender.createMimeMessage();
MimeMessageHelper messageHelper = new MimeMessageHelper(message, true, "UTF-8");
messageHelper.setFrom("[email protected]");
messageHelper.setTo("[email protected]");
messageHelper.setSubject("Spring mail test");
messageHelper.setText("Plain message", "<html><body><h2>html message</h2></body></html>");
javaMailSender.send(message);
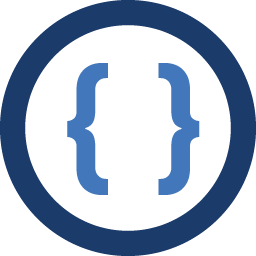
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I'd like to prepare simple html email with alternative plain-text version. I don't need any attachments or inline elements.
By default if I use:
MimeMessageHelper message = new MimeMessageHelper(mimeMessage, true, "UTF-8");
I get
MimeMessageHelper.MULTIPART_MODE_MIXED_RELATED
mode.My email content body looks like this:
Content-Type: multipart/mixed; boundary="----=_Part_8_21489995.1282317482209" ------=_Part_8_21489996.1282317482209 Content-Type: multipart/related; boundary="----=_Part_9_21489996.1282317482209" ------=_Part_9_21489996.1282317482209 Content-Type: multipart/alternative; boundary="----=_Part_10_2458205.1282317482209" ------=_Part_10_2458205.1282317482209 Content-Type: text/plain; charset=UTF-8 Content-Transfer-Encoding: quoted-printable Simple newsletter. ------=_Part_10_2458205.1282317482209 Content-Type: text/html;charset=UTF-8 Content-Transfer-Encoding: quoted-printable <html> <head> <title>Simple newsletter</title> <head> <body> <p>Simple newsletter.</p> </body> <html> ------=_Part_10_2458205.1282317482209-- ------=_Part_9_21489996.1282317482209-- ------=_Part_8_21489995.1282317482209--
What can I do to get rid of mixed and related boundries?
Ideal solution would be
MimeMessageHelper.MULTIPART_MODE_ALTERNATIVE
mode, but it's not available.