Problem when assigning a pointer to an enum variable in C
Solution 1
Because status is a pointer to enum error_type, and the_go_status is a pointer to an int. They are pointers to different types.
Solution 2
I'm not sure if this is related exactly to your warning or not, but be very careful assigning references to local variables to pointers within structs. If the_go_status
is a local, as soon as your function returns, the reference to that local will become invalid. So, if your code (or someone else's code) uses your instance of error_struct
outside the function declaring the_go_status
, things will quickly break.
Solution 3
This is because enum error_type *
isn't compatible with int *
, because they point to values of different types (and possibly even different sizes). You should declare the_go_status
as:
enum error_type the_go_status;
Although simply casting the pointer (i.e. (enum error_type *)&the_go_status
) will make the warning go away, it may result in bugs on some platforms. See Is the sizeof(enum) == sizeof(int), always?
Solution 4
you should declare a pointer if you want to use a pointer:
int * the_go_status
otherwise you declare a primitive, which is not placed on the heap, but on the stack. (please correct my when being wrong on that)
However, I don't get why you want to use a pointer at all. Just do something like this in your struct definition:
enum error_type status;
and change your last line to:
error_struct.status = the_go_status;
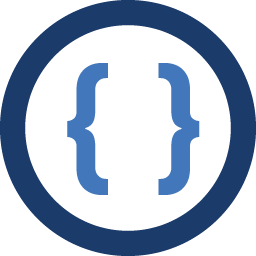
Admin
Updated on June 22, 2022Comments
-
Admin almost 2 years
I am getting a warning of "assigment from incompatible pointer type". I don't understand why this warning is happening. I don't know what else to declare "the_go_status" variable to other than an integer. (Note: this is not all the code, but just a simplified version I posted to illustrate the problem.)
The warning occurs on the last line of the example I included below.
//In a header file enum error_type { ERR_1 = 0, ERR_2 = 1, ERR_3 = 2, ERR_4 = 4, }; //In a header file struct error_struct { int value; enum error_type *status; }; //In a C file int the_go_status; the_go_status = ERR_1; //Have the error_struct "status" point to the address of "the_go_status" error_struct.status = &the_go_status; //WARNING HERE!