Problem with http.get and Null types in response
I am not sure how are you importing the http package. If you are using "http" alias to call get() make sure you import as follows,
import 'package:http/http.dart' as http;
and also use the Response class from the package as
http.Response;
I tried to get the data from the given url, it worked for me.
...
import 'package:http/http.dart' as http;
...
Future<void> fetchData() async {
const url = "http://www.wienerlinien.at/ogd_realtime/monitor?&stopId=4133";
final http.Response response = await http.get(url);
final data = json.decode(response.body) as Map<String, dynamic>;
print(data);
}
Alternatively you can skip the class and let Dart infer the response type as follows:
...
import 'package:http/http.dart' as http;
...
Future<void> fetchData() async {
const url = "http://www.wienerlinien.at/ogd_realtime/monitor?&stopId=4133";
final response = await http.get(url);
final data = json.decode(response.body) as Map<String, dynamic>;
print(data);
}
Debug Console:
I/flutter (20609): {data: {monitors: [{locationStop: {type: Feature, geometry: {type: Point, coordinates: [null, null]}, properties: {name: 60200949, title: Oberlaa, municipality: Wien, municipalityId: 90001, type: stop, coordName: WGS84, gate: 2, attributes: {rbl: 4133}}}, lines: [{name: U1, towards: NICHT EINSTEIGEN ! NO DEPARTURE PLATFORM, direction: R, platform: 2, richtungsId: 2, barrierFree: true, realtimeSupported: true, trafficjam: false, departures: {departure: [{departureTime: {}, vehicle: {name: U1, towards: NICHT EINSTEIGEN ! NO DEPARTURE PLATFORM, direction: R, richtungsId: 2, barrierFree: false, realtimeSupported: true, trafficjam: false, type: ptMetro, attributes: {}, linienId: 301}}]}, type: ptMetro, lineId: 301}], attributes: {}}]}, message: {value: OK, messageCode: 1, serverTime: 2020-07-19T15:55:30.000+0200}}
You can then do null checking on the data. Also try putting code in a try catch block wherever you are calling your fetchData() funtion to handle errors properly.
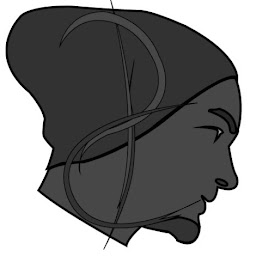
Chris Pi
Updated on December 22, 2022Comments
-
Chris Pi over 1 year
Im working on a project with the OGD of the Austrian public transportation API which wants for a request just a getter with a "stopID". Also you can concatenate more than one stopID. That means if you try this link, you get a response with all data of the stopIDs:
And now here is my problem: The stopID with the number 4133 (try it on http://www.wienerlinien.at/ogd_realtime/monitor?&stopId=4133) has NULL values in the coordinates. Normally I would just do some NULL checks but there is some strange behaviour I've never seen. I've debugged to the point of the error. It's at the HTTP.get Request and says:
I/flutter (29464): ERROR: Invalid argument(s) (input): Must not be null
But how is that possible if I havent gotten the response at this point? It tries to request and breaks somewhere at building the response.
This is the request code for it (
finalURl
is the URL from above):final Response response = await http.get(finalUrl); if (response.statusCode == 200) { final encode = jsonDecode(response.body); }
The error happens even before the
if
statement and parsing the json String. So before it gets a proper Response Object. If you ask now how I know why it happens because of the NULL types in the coordinate fields, I've tried the Request without the ID 4133 and it worked fine. If I just use the second request Link (with only the ID 4133), it throws the error. Does anybody have an idea whats wrong there? It is definetly a problem with Dart/Flutter, did I miss something?-
julemand101 almost 4 yearsCan you please attach the full stacktrace?
-
Chris Pi almost 4 years@julemand101 Thats the weird part. There is no stacktrace, just this line pops up and the app stops running.
-
julemand101 almost 4 yearsCan you make a small example which reproduce the issue which you can attach to your question?
-
-
Chris Pi almost 4 yearsYeah, that will work for sure. My problem is, that the error comes earlier before i can check if the value is null. Even before the json parsing happens
-
Chris Pi almost 4 yearsI found the problem after 2 hours getting over all. The problem was not the get request, the problem was my model, which handled the departure time wrong at empty departure lists. However, it gets me that the debugger stopped everytime after the get request and never passed the if statement to check the statusCode
-
Ibrahim Broachwala almost 4 yearsGlad you found the solution :D