Process argc and argv outside of main()
Solution 1
Either pass them as parameters, or store them in global variables. As long as you don't return from main and try to process them in an atexit
handler or the destructor of an object at global scope, they still exist and will be fine to access from any scope.
For example:
// Passing them as args:
void process_command_line(int argc, char **argv)
{
// Use argc and argv
...
}
int main(int argc, char **argv)
{
process_command_line(argc, argv);
...
}
Alternatively:
// Global variables
int g_argc;
char **g_argv;
void process_command_line()
{
// Use g_argc and g_argv
...
}
int main(int argc, char **argv)
{
g_argc = argc;
g_argv = argv;
process_command_line();
...
}
Passing them as parameters is a better design, since it's encapsulated and let's you modify/substitute parameters if you want or easily convert your program into a library. Global variables are easier, since if you have many different functions which access the args for whatever reason, you can just store them once and don't need to keep passing them around between all of the different functions.
Solution 2
One should keep to standards wherever practical. Thus, don't write
void main
which has never been valid C or C++, but instead write
int main
With that, your code can compile with e.g. g++
(with usual compiler options).
Given the void main
I suspect a Windows environment. And anyway, in order to support use of your program in a Windows environment, you should not use the main
arguments in Windows. They work in *nix because they were designed in and for that environment; they don't in general work in Windows, because by default (by very strong convention) they're encoded as Windows ANSI, which means they cannot encode filenames with characters outside the user's current locale.
So for Windows you better use the GetCommandLine
API function and its sister parsing function. For portability this should better be encapsulated in some command line arguments module. Then you need to deal with the interesting problem of using wchar_t
in Windows and char
in *nix…
Anyway, I'm not sure of corresponding *nix API, or even if there is one, but google it. In the worst case, for *nix you can always initialize a command line arguments module from main
. The ugliness for *nix stems directly from the need to support portability with C++'s most non-portable, OS-specific construct, namely standard main
.
Solution 3
Simply pass argc
and argv
as arguments of the function in which you want to process them.
void parse_arg(int argc, char *argv[]);
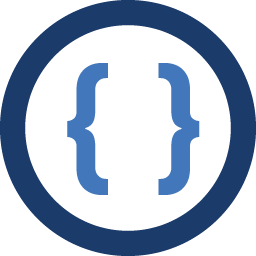
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
If I want to keep the bulk of my code for processing command line arguments out of main (for organization and more readable code), what would be the best way to do it?
void main(int argc, char* argv[]){ //lots of code here I would like to move elsewhere }
-
Jonathan Leffler almost 8 yearsI whole-heartedly agree with
int main
overvoid main
. However, the other commentary leaves me … bemused. Which system other than Windows has a problem with the standard C++ convention formain()
? I'm not aware of one, so castigating the standard convention as 'non-portable' and 'OS-specific' seems OTT. -
Cheers and hth. - Alf almost 8 years@JonathanLeffler: I'm not aware of any commonly used system other than Windows where the
main
arguments don't work (other than for English alphabet pure ASCII text). Still, a feature that doesn't work in Windows is non-portable, and a feature designed for Unix-land is OS-specific. And themain
arguments convention both doesn't work in Windows (i.e. is non-portable), and is designed for Unix-land (i.e. is OS-specific), and those two aspects are strongly connected. There's absolutely nothing amusing about it. I can only think of ugly political reasons for its persistence.