Programmatically define execution order of scripts
Solution 1
Short answer: No. But you can set Script Execution Order in the settings (menu: Edit > Project Settings > Script Execution Order) or change it from code:
// First you get the MonoScript of your MonoBehaviour
MonoScript monoScript = MonoScript.FromMonoBehaviour(yourMonoBehaviour);
// Getting the current execution order of that MonoScript
int currentExecutionOrder = MonoImporter.GetExecutionOrder(monoScript);
// Changing the MonoScript's execution order
MonoImporter.SetExecutionOrder(monoScript, x);
1) Script Execution Order manipulation
2) Changing Unity Scripts Execution Order from Code
3) Change a script's execution order dynamically (from script)
Recently I've also faced similar issue, and found this question without an answer. Thus decided to post useful information, hope it helps :)
Solution 2
you can use the attribute:
[DefaultExecutionOrder(100)]
public class SomeClass : MonoBehaviour
{
}
Solution 3
Some of the assets I've seen in the store, for example Cinemachine, set the executionOrder property in the .meta file (for example, MyMonoBehavior.cs.meta). I've tested and this indeed works (for example, change it from 0 to 200 then right-click and Reimport). It seems that the meta files are preserved in the package, so if you are distributing your asset, putting your asset package in the store with meta should work (https://docs.unity3d.com/Manual/AssetPackages.html).
Solution 4
As mentioned here
By default, the Awake, OnEnable and Update functions of different scripts are called in the order the scripts are loaded (which is arbitrary). However, it is possible to modify this order using the Script Execution Order settings.
Reference to Execution Order Setting can be found here
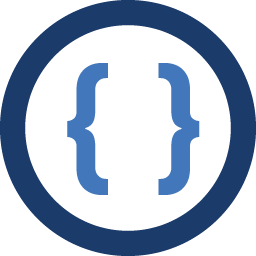
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
By programmatically adding scripts to a given game object, will these scripts execute in the order they were added? Will their events run in the order they were added?
void Awake () { gameObject.AddComponent("Script_1"); gameObject.AddComponent("Script_2"); }
-
Admin over 9 yearsRight, but that doesn't answer my question which asks about non-arbitrary loading (non-random). So, loading scripts programmatically via the awake, are they run in the order they are added and continue to run in that order?
-
maZZZu over 9 yearsI think that you cannot get any better answer than that. It is not defined in which order they are executed. Unless you modify the order using the Script Execution Order Settings. The execution order is not defined probably because unity is executing them with multiple threads. After finishing with previous function each thread probably just processes next function that is not yet started to process.
-
Bip901 almost 5 yearsThis is exactly what I was looking for. Thank you very much!
-
Bilal Akil about 4 yearsAwesome! In case you're worried this isn't doing anything (as I was) because it doesn't show up in the Script Execution Order window, that's apparently by design: forum.unity.com/threads/…
-
zezba9000 about 3 yearsAnd your 'yourMonoBehaviour' handle comes from where? You allocate a new one? This just returns null.
-
gresolio about 3 yearsTypically you are doing such things from an Editor script, so you can cast your target to MonoBehaviour:
MonoBehaviour yourMonoBehaviour = (MonoBehaviour)target;
If you are not familiar with Editor scripting, take a look some tutorials, e.g. learn.unity.com/tutorial/editor-scripting -
zezba9000 about 3 yearsic tnx. Also found '[DefaultExecutionOrder(100)]' to be a better solution for my case.