Programmatically set filename and path in Microsoft Print to PDF printer
Solution 1
As noted in other answers, you can force PrinterSettings.PrintToFile = true
, and set the PrinterSettings.PrintFileName
, but then your user doesn't get the save as popup. My solution is to go ahead and show the Save As dialog myself, populating that with my "suggested" filename [in my case, a text file (.txt) which I change to .pdf], then set the PrintFileName
to the result.
DialogResult userResp = printDialog.ShowDialog();
if (userResp == DialogResult.OK)
{
if (printDialog.PrinterSettings.PrinterName == "Microsoft Print to PDF")
{ // force a reasonable filename
string basename = Path.GetFileNameWithoutExtension(myFileName);
string directory = Path.GetDirectoryName(myFileName);
prtDoc.PrinterSettings.PrintToFile = true;
// confirm the user wants to use that name
SaveFileDialog pdfSaveDialog = new SaveFileDialog();
pdfSaveDialog.InitialDirectory = directory;
pdfSaveDialog.FileName = basename + ".pdf";
pdfSaveDialog.Filter = "PDF File|*.pdf";
userResp = pdfSaveDialog.ShowDialog();
if (userResp != DialogResult.Cancel)
prtDoc.PrinterSettings.PrintFileName = pdfSaveDialog.FileName;
}
if (userResp != DialogResult.Cancel) // in case they canceled the save as dialog
{
prtDoc.Print();
}
}
Solution 2
I did some experimenting myself but like yourself was also not able to prefill the SaveAs dialog in the PrintDialog with the DocumentName or PrinterSettings.PrintFileName already filled in the PrintDocument instance. This seems counterintuitive, so maybe I'm missing something
If you want to, you can however bypass the printdialog and print automatically without any user interaction at all. If you choose to do so, you must make sure beforehand that the directory to which you want to add a document to is in existence and that the document to be added is not in existence.
string existingPathName = @"C:\Users\UserName\Documents";
string notExistingFileName = @"C:\Users\UserName\Documents\TestPrinting1.pdf";
if (Directory.Exists(existingPathName) && !File.Exists(notExistingFileName))
{
PrintDocument pdoc = new PrintDocument();
pdoc.PrinterSettings.PrinterName = "Microsoft Print to PDF";
pdoc.PrinterSettings.PrintFileName = notExistingFileName;
pdoc.PrinterSettings.PrintToFile = true;
pdoc.PrintPage += pdoc_PrintPage;
pdoc.Print();
}
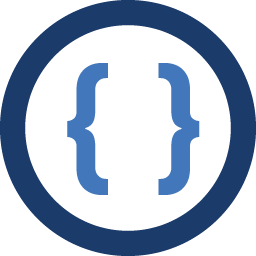
Admin
Updated on December 01, 2020Comments
-
Admin over 3 years
I have a
C# .net
program that creates various documents. These documents should be stored in different locations and with different, clearly defined names.To do so, I use the
System.Drawing.Printing.PrintDocument
class. I select theMicrosoft Print to PDF
as printer with this statement:PrintDocument.PrinterSettings.PrinterName = "Microsoft Print to PDF"
;While doing so I'm able to print my document in a
pdf file
. The user gets a file select dialog. He can then specify in this dialog box the name of the pdf file and where to store it.As the amount of files are large and it is annoying and error-prone to find always the correct path and name, I would like to set the correct path and filename in this dialog box programmatically.
I have already tested these attributes:
PrintDocument.PrinterSettings.PrintFileName PrintDocument.DocumentName
Writing the required path and filename to these attributes didn't help. Does anybody know, how to set the default values for path and filename for the
Microsoft Print to PDF
printer in C#?Note: My environment : Windows 10, Visual Studio 2010, .net framework 4.5