Properly formatted multiplication table
Solution 1
Quick way (Probably too much horizontal space though):
n=int(input('Please enter a positive integer between 1 and 15: '))
for row in range(1,n+1):
for col in range(1,n+1):
print(row*col, end="\t")
print()
Better way:
n=int(input('Please enter a positive integer between 1 and 15: '))
for row in range(1,n+1):
print(*("{:3}".format(row*col) for col in range(1, n+1)))
And using f-strings (Python3.6+)
for row in range(1, n + 1):
print(*(f"{row*col:3}" for col in range(1, n + 1)))
Solution 2
Gnibbler's approach is quite elegant. I went for the approach of constructing a list of list of integers first, using the range function and taking advantage of the step argument.
for n = 12
import pprint
n = 12
m = list(list(range(1*i,(n+1)*i, i)) for i in range(1,n+1))
pprint.pprint(m)
[[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12],
[2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24],
[3, 6, 9, 12, 15, 18, 21, 24, 27, 30, 33, 36],
[4, 8, 12, 16, 20, 24, 28, 32, 36, 40, 44, 48],
[5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60],
[6, 12, 18, 24, 30, 36, 42, 48, 54, 60, 66, 72],
[7, 14, 21, 28, 35, 42, 49, 56, 63, 70, 77, 84],
[8, 16, 24, 32, 40, 48, 56, 64, 72, 80, 88, 96],
[9, 18, 27, 36, 45, 54, 63, 72, 81, 90, 99, 108],
[10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 110, 120],
[11, 22, 33, 44, 55, 66, 77, 88, 99, 110, 121, 132],
[12, 24, 36, 48, 60, 72, 84, 96, 108, 120, 132, 144]]
Now that we have a list of list of integers that is in the form that we want,
we should convert them into strings that are right justified with a width
of one larger than the largest integer in the list of lists (the last integer),
using the default argument of ' '
for the fillchar.
max_width = len(str(m[-1][-1])) + 1
for i in m:
i = [str(j).rjust(max_width) for j in i]
print(''.join(i))
1 2 3 4 5 6 7 8 9 10 11 12
2 4 6 8 10 12 14 16 18 20 22 24
3 6 9 12 15 18 21 24 27 30 33 36
4 8 12 16 20 24 28 32 36 40 44 48
5 10 15 20 25 30 35 40 45 50 55 60
6 12 18 24 30 36 42 48 54 60 66 72
7 14 21 28 35 42 49 56 63 70 77 84
8 16 24 32 40 48 56 64 72 80 88 96
9 18 27 36 45 54 63 72 81 90 99 108
10 20 30 40 50 60 70 80 90 100 110 120
11 22 33 44 55 66 77 88 99 110 121 132
12 24 36 48 60 72 84 96 108 120 132 144
and demonstrate the elasticity of the spacing with a different size, e.g. n = 9
n=9
m = list(list(range(1*i,(n+1)*i, i)) for i in range(1,n+1))
for i in m:
i = [str(j).rjust(len(str(m[-1][-1]))+1) for j in i]
print(''.join(i))
1 2 3 4 5 6 7 8 9
2 4 6 8 10 12 14 16 18
3 6 9 12 15 18 21 24 27
4 8 12 16 20 24 28 32 36
5 10 15 20 25 30 35 40 45
6 12 18 24 30 36 42 48 54
7 14 21 28 35 42 49 56 63
8 16 24 32 40 48 56 64 72
9 18 27 36 45 54 63 72 81
Solution 3
for i in range(1, 10) :
for j in range(1, 10):
print(repr(i*j).rjust(4),end=" ")
print()
print()
Output:
1 2 3 4 5 6 7 8 9
2 4 6 8 10 12 14 16 18
3 6 9 12 15 18 21 24 27
4 8 12 16 20 24 28 32 36
5 10 15 20 25 30 35 40 45
6 12 18 24 30 36 42 48 54
7 14 21 28 35 42 49 56 63
8 16 24 32 40 48 56 64 72
9 18 27 36 45 54 63 72 81
or this one
for i in range(1, 11):
for j in range(1, 11):
print(("{:6d}".format(i * j,)), end='')
print()
the result is :
1 2 3 4 5 6 7 8 9 10
2 4 6 8 10 12 14 16 18 20
3 6 9 12 15 18 21 24 27 30
4 8 12 16 20 24 28 32 36 40
5 10 15 20 25 30 35 40 45 50
6 12 18 24 30 36 42 48 54 60
7 14 21 28 35 42 49 56 63 70
8 16 24 32 40 48 56 64 72 80
9 18 27 36 45 54 63 72 81 90
10 20 30 40 50 60 70 80 90 100
Solution 4
Creating Arithmetic table is much simpler but i thought i should post my answer despite the fact there are so many answers to this question because no one talked about limit of table.
Taking input from user as an integer
num = int(raw_input("Enter your number"))
Set limit of table, to which extent we wish to calculate table for desired number
lim = int(raw_input("Enter limit of table"))
Iterative Calculation starting from index 1
In this, i've make use of slicing with format to adjust whitespace between number i.e., {:2} for two space adjust.
for b in range(1, lim+1):
print'{:2} * {:2} = {:2}'.format(a, b, a*b)
Final CODE:
num = int(raw_input("Enter your number"))
lim = int(raw_input("Enter limit of table"))
for b in range(1, lim+1):
print'{:2} * {:2} = {:2}'.format(a, b, a*b)
OUTPUT:
Enter your number 2
Enter limit of table 20
2 * 1 = 2
2 * 2 = 4
2 * 3 = 6
2 * 4 = 8
2 * 5 = 10
2 * 6 = 12
2 * 7 = 14
2 * 8 = 16
2 * 9 = 18
2 * 10 = 20
2 * 11 = 22
2 * 12 = 24
2 * 13 = 26
2 * 14 = 28
2 * 15 = 30
2 * 16 = 32
2 * 17 = 34
2 * 18 = 36
2 * 19 = 38
2 * 20 = 40
Solution 5
Or you could just do this (not as simplistic as the others but it works):
def main():
rows = int(input("Enter the number of rows that you would like to create a multiplication table for: "))
counter = 0
multiplicationTable(rows,counter)
def multiplicationTable(rows,counter):
size = rows + 1
for i in range (1,size):
for nums in range (1,size):
value = i*nums
print(value,sep=' ',end="\t")
counter += 1
if counter%rows == 0:
print()
else:
counter
main()
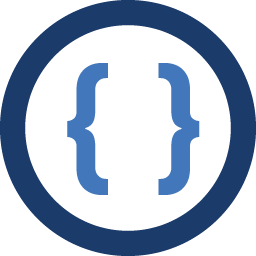
Admin
Updated on January 02, 2022Comments
-
Admin over 2 years
How would I make a multiplication table that's organized into a neat table? My current code is:
n=int(input('Please enter a positive integer between 1 and 15: ')) for row in range(1,n+1): for col in range(1,n+1): print(row*col) print()
This correctly multiplies everything but has it in list form. I know I need to nest it and space properly, but I'm not sure where that goes?
-
Adam Smith over 10 yearsWhat's going on in that print function -- can you elaborate?
-
Pitarou over 10 yearsThe (... for ... in ...) generates an iterable. The *(... for ... in ...) turns the iterable into a list of arguments to supply to print. See my answer for a discussion of how the print works. It's a fairly advanced trick, so don't worry if you don't understand it.
-
martineau over 10 years@adsmith:
print()
is a built-in function and the output string is being formatted using a format specification that's part of a generator expression. -
mikus over 8 yearsare you sure it adds any value to the below answers? I'd say it's far less clean.
-
Yehuda Katz over 8 yearsI appreciate your comment. Yehuda
-
Joey Baruch about 8 yearsi get a "Syntax error while detecting tuple" on your Better way solution.
-
John La Rooy about 8 years@joeybaruch, are you using Python2? The question is tagged Python-3.x
-
James K over 7 yearsYour answer is very short and lacks context. When you have a little more reputation, you will be able to suggest improvements using comments.
-
abhinav about 7 yearsyour code is much simpler but. it would be better if you explain a little bit further.
-
Admin about 7 years@Abhinav Thx for urs insight.
-
Charnel about 4 yearsHi! Thanks for sharing answer but could you please correct the code formatting?
-
Sunshine about 4 yearsSure, what do you want me to do? Get rid of the empty lines/spaces?
-
sancelot about 4 yearsplease can you give more details about this piece of code, and what was wrong in the question asked.
-
Yehuda Katz almost 4 years... and yes, I'm sure.