Pure JavaScript instead of jQuery - ready, on, click and end selector
10,076
Solution 1
Use addEventListener
instead of on
:
document.addEventListener('DOMContentLoaded', function () {
document.body.addEventListener('click', function (e) {
if (e.target.matches("[id$='someId']")) {
console.log(e.target.value);
}
});
});
The event delegation makes it a bit more complex, but it should do the trick.
Solution 2
$(document).ready(function()
// DOMContentLoaded
// @see https://developer.mozilla.org/en-US/docs/Web/Events/DOMContentLoaded
document.addEventListener('DOMContentLoaded', function (event) {
console.log('DOM fully loaded and parsed');
});
$(document).on
document.addEventListener("click", function(event) {
var target = event.target;
if (target.id === 'a') {
// do something.
} else if (target.id === 'b') {
// do something.
}
});
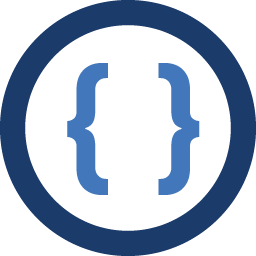
Author by
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I have in jQuery:
$(document).ready(function() { $(document).on('click', "[id$='someId']", function () { console.log($(this).val()); }) })
How can I write it in pure JavaScript?
$(document).ready(function()
Should I use "ready" in pure JavaScript?
$(document).on
How can I use "on"? I have to add dynamic elements, so in jQuery I must use "on".
I would like write this in accordance with ES6.