PyQt Open File Dialog - Display Path Name
16,083
First you need to create a TextEdit object, like self.myTextBox = QtGui.QTextEdit(self)
. After that place it wherever you want it on the screen. Then you need to connect it with your open
function. It should look something like this.
def open(self):
fileName = QtGui.QFileDialog.getOpenFileName(self, 'OpenFile')
self.myTextBox.setText(fileName)
print(fileName)
This will display the path of chosen file on your textBox
.
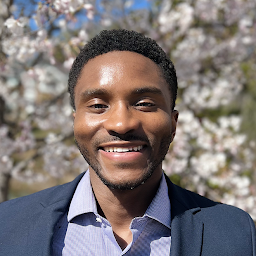
Author by
olanre
Updated on June 05, 2022Comments
-
olanre almost 2 years
Using PyQt, I have created a button that can choose and upload a file from any directory. How do I create a text box next to the button that would display the path of the file selected or opened?
Here is my sample code:
self.uploadButton = QtGui.QPushButton('UPLOAD SDF', self) self.runfilterButton = QtGui.QPushButton('Run Filter', self) self.printimagesButton = QtGui.QPushButton('Display Matches Images', self) self.listmatchesButton = QtGui.QPushButton('List Matches', self) self.uploadButton.move (100, 50) self.runfilterButton.move (400,50) self.printimagesButton.move (200, 100) self.printimagesButton.resize (200, 50) self.listmatchesButton.move (200, 150) self.listmatchesButton.resize (200, 50) hBoxLayout = QtGui.QHBoxLayout() hBoxLayout.addWidget(self.uploadButton) hBoxLayout.addWidget(self.runfilterButton) self.setLayout(hBoxLayout) # Signal Init. self.connect(self.uploadButton, QtCore.SIGNAL('clicked()'), self.open) self.runfilterButton.clicked.connect(runfilterx) self.printimagesButton.clicked.connect(printimages) self.listmatchesButton.clicked.connect(listmatches)