Python 2.7 creating a multidimensional list
Solution 1
I think your list comprehension versions were very close to working. You don't need to do any list multiplication (which doesn't work with empty lists anyway). Here's a working version:
>>> y = [[[] for i in range(n)] for i in range(n)]
>>> print y
[[[], [], [], []], [[], [], [], []], [[], [], [], []], [[], [], [], []]]
Solution 2
looks like the most easiest way is as follows:
def create_empty_array_of_shape(shape):
if shape: return [create_empty_array_of_shape(shape[1:]) for i in xrange(shape[0])]
it's work for me
Solution 3
A very simple and elegant way is:
a = [([0] * 5) for i in range(5)]
a
[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]]
Solution 4
In Python I made a little factory method to create a list of variable dimensions and variable sizes on each of those dimensions:
def create_n_dimensional_matrix(self, n):
dimensions = len(n)
if (dimensions == 1):
return [0 for i in range(n[0])]
if (dimensions == 2):
return [[0 for i in range(n[0])] for j in range(n[1])]
if (dimensions == 3):
return [[[0 for i in range(n[0])] for j in range(n[1])] for k in range(n[2])]
if (dimensions == 4):
return [[[[0 for i in range(n[0])] for j in range(n[1])] for k in range(n[2])] for l in range(n[3])]
run it like this:
print(str(k.create_n_dimensional_matrix([2,3])))
print(str(k.create_n_dimensional_matrix([3,2])))
print(str(k.create_n_dimensional_matrix([1,2,3])))
print(str(k.create_n_dimensional_matrix([3,2,1])))
print(str(k.create_n_dimensional_matrix([2,3,4,5])))
print(str(k.create_n_dimensional_matrix([5,4,3,2])))
Which prints:
- The two dimensional lists (2x3), (3x2)
- The three dimensional lists (1x2x3),(3x2x1)
-
The four dimensional lists (2x3x4x5),(5x4x3x2)
[[0, 0], [0, 0], [0, 0]] [[0, 0, 0], [0, 0, 0]] [[[0], [0]], [[0], [0]], [[0], [0]]] [[[0, 0, 0], [0, 0, 0]]] [[[[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]]], [[[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]]], [[[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]]], [[[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]]], [[[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]], [[0, 0], [0, 0], [0, 0]]]] [[[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]], [[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]], [[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]]], [[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]], [[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]], [[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]]]]
Solution 5
i found this:
Matrix = [[0 for x in xrange(5)] for x in xrange(5)]
You can now add items to the list:
Matrix[0][0] = 1
Matrix[4][0] = 5
print Matrix[0][0] # prints 1
print Matrix[4][0] # prints 5
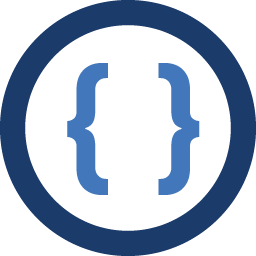
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
In Python I want an intuitive way to create a 3 dimensional list.
I want an (n by n) list. So for n = 4 it should be:
x = [[[],[],[],[]],[[],[],[],[]],[[],[],[],[]],[[],[],[],[]]]
I've tried using:
y = [n*[n*[]]] y = [[[]]* n for i in range(n)]
Which both appear to be creating copies of a reference. I've also tried naive application of the list builder with little success:
y = [[[]* n for i in range(n)]* n for i in range(n)] y = [[[]* n for i in range(1)]* n for i in range(n)]
I've also tried building up the array iteratively using loops, with no success. I also tried this:
y = [] for i in range(0,n): y.append([[]*n for i in range(n)])
Is there an easier or more intuitive way of doing this?
-
Blckknght over 11 yearsThat was mine, from before your edits. I've removed it now, since your answer is at least sensible now. I think it's still technically wrong, since the questioner specifically wanted an
n
byn
by0
three dimensional structure and you're only making ann
byn
two dimensional one. -
user1505695 over 11 yearsfair enough. but this answer can be easily extended to 3D, no? or maybe i misinterpreted the question.
-
pterodragon over 8 yearsI'm not sure if SO should handle cross-links smarter :)
-
DavidW over 8 yearsPossibly. I've deleted my comment anyway since it no longer applies... :)
-
orange over 8 yearsSee user2114402's answer. That's a generic way post more than 2 years before your's. "I am amazed that no one" reads previously posted answers ;-).
-
pterodragon over 8 yearswhoops...missed that :D. I originally posted the answer for the other question and I copied that here. Upvoted user2114402's for the short recursive answer.
-
Vladimir about 8 yearsI am interested in an even more generic answer to that question. Imagine we have N dimensions and we need to both get and set an element. Getting an element will be a relatively simple recursion, while setting an element is not that easy (correct me if I am wrong). "l[1][1][1]" won't work simply because we don't know the number of dimensions in advance.
-
pterodragon about 8 yearsI don't quite get exactly what you want. You assumed that the list is an N (known) dimensional list and said you can't set the element in the list because N is unknown?