Python assert isinstance() Vector
assert
is better used for debugging than left loafing around in production code. You can instead create properties for the vector attributes x
, y
and z
, and raise ValueError
when the passed values are not of the required type:
class Vector3:
def __init__(self, x=0.0, y=0.0, z=0.0):
self.x = x
self.y = y
self.z = z
@property
def x(self):
return self._x
@x.setter
def x(self, val):
if not isinstance(val, (int, float)):
raise TypeError('Inappropriate type: {} for x whereas a float \
or int is expected'.format(type(val)))
self._x = float(val)
...
Notice how isinstance
also takes a tuple of types.
In the __add__
operator, you'll want to raise TypeError
also, including an appropriate message:
def __add__(self, other):
if not isinstance(other, Vector3):
raise TypeError('Object of type Vector3 expected, \
however type {} was passed'.format(type(other)))
...
Related videos on Youtube
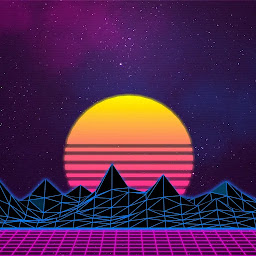
Stefan B
Updated on July 17, 2021Comments
-
Stefan B almost 3 years
I am trying to implement a Vector3 class in python. If I would write the Vector3 class in c++ or c# i would have X, Y, and Z members stored as floats but in python I read that ducktyping is the way to go. So according to my c++/c# knowledge I wrote something like this:
class Vector3: def __init__(self, x=0.0, y=0.0, z=0.0): assert (isinstance(x, float) or isinstance(x, int)) and (isinstance(y, float) or isinstance(y, int)) and \ (isinstance(z, float) or isinstance(z, int)) self.x = float(x) self.y = float(y) self.z = float(z)
The question is regarding the assert statements: Would you use them or not in this instance (a Vector3 implementation for math). I also used it for the operations like
def __add__(self, other): assert isinstance(other, Vector3) return Vector3(self.x + other.x, self.y + other.y, self.z + other.z)
Would you use assert in these instances or not? According to this website : https://wiki.python.org/moin/UsingAssertionsEffectively it should not be overused but for me as someone who used static typing all along, not checking for the same datatype is extremely weird.
-
Stefan B over 6 yearsSo You would also use isinstance() in that example and not just expect the right type right away?
-
Moses Koledoye over 6 years@StefanB You can, to prevent users from passing other numeric types such as complex types.