Python AttributeError: class object has no attribute
12,846
Solution 1
Martijn's answer explains the problem and gives the minimal solution. However, given that self.markers
appears to be constant, I would make it a class attribute rather than recreating it for every instance:
class TTYFigureData(object):
"""Data container of TTYFigure."""
MARKERS = {
"-": u"None" ,
",": u"\u2219",
}
def __init__(self, x, y, marker='_.', plot_slope=True):
"""Document parameters here as required."""
self.x = x
self.y = y
self.plot_slope = plot_slope
self.set_marker(marker)
def set_marker(self, marker):
"""See also here - usage guidance is also good."""
if marker in [None, "None", u"None", ""]:
self.plot_slope = True
self.marker = ""
elif marker[0] == "_":
self.marker = self.MARKERS[marker[1:]]
else:
self.marker = marker
(note also changes to style in line with the official guidance)
Solution 2
In your __init__
method, you call self.set_marker()
before you set self.markers
:
self.set_marker(marker)
self.markers = {
"-" : u"None" ,
"," : u"\u2219"
}
So when set_marker()
runs, there is no self.markers
yet. Move the call down a line:
self.markers = {
"-" : u"None" ,
"," : u"\u2219"
}
self.set_marker(marker)
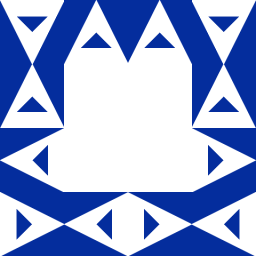
Author by
d3pd
Updated on June 04, 2022Comments
-
d3pd 12 months
When I try to run the code of a class I'm writing, I get an
AttributeError
and I'm not sure why. The specific error is as follows:self.marker = self.markers[marker[1:]] AttributeError: 'TTYFigureData' object has no attribute 'markers'
Here is part of the class I'm writing:
class TTYFigureData(object): """ data container of TTYFigure """ def __init__( self, x, # x values y, # y values marker = "_.", # datum marker plot_slope = True ): self.x = x self.y = y self.plot_slope = plot_slope self.set_marker(marker) self.markers = { "-" : u"None" , "," : u"\u2219" } def set_marker( self, marker ): if marker in [None, "None", u"None", ""]: self.plot_slope = True self.marker = "" elif marker[0] == "_": self.marker = self.markers[marker[1:]] else: self.marker = marker
Where am I going wrong?