Python: Check if any file exists in a given directory
15,696
To only check one specific directory, a solution like this would suffice:
from os import listdir
from os.path import isfile, join
def does_file_exist_in_dir(path):
return any(isfile(join(path, i)) for i in listdir(path))
To dissect what is happening:
- The method
does_file_exist_in_dir
will take your path. - Using any it will return True if a file is found by iterating through the contents of your path by calling a listdir on it. Note the use of join for the path in order to provide a qualified filepath name to properly check.
As an option, if you want to traverse through all sub-directories of a given path and check for files, you can use os.walk and just check to see if the level you are in contains any files like this:
for dir, sub_dirs, files in os.walk(path):
if not files:
print("no files at this level")
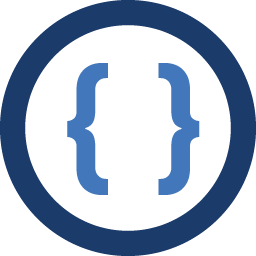
Author by
Admin
Updated on July 29, 2022Comments
-
Admin almost 2 years
Given a directory as a string, how can I find if ANY file exists in it?
os.path.isFile() # only accepts a specific file path os.listdir(dir) == [] # accepts sub-directories
My objective is to check if a path is devoid of files only (not sub-directories too).