Python Check if mouse clicked
Solution 1
I was able to make it work just with win32api. It works when clicking on any window.
import win32api
import time
width = win32api.GetSystemMetrics(0)
height = win32api.GetSystemMetrics(1)
midWidth = int((width + 1) / 2)
midHeight = int((height + 1) / 2)
state_left = win32api.GetKeyState(0x01) # Left button up = 0 or 1. Button down = -127 or -128
while True:
a = win32api.GetKeyState(0x01)
if a != state_left: # Button state changed
state_left = a
print(a)
if a < 0:
print('Left Button Pressed')
else:
print('Left Button Released')
win32api.SetCursorPos((midWidth, midHeight))
time.sleep(0.001)
Solution 2
Try this
from pynput.mouse import Listener
def on_move(x, y):
print(x, y)
def on_click(x, y, button, pressed):
print(x, y, button, pressed)
def on_scroll(x, y, dx, dy):
print(x, y, dx, dy)
with Listener(on_move=on_move, on_click=on_click, on_scroll=on_scroll) as listener:
listener.join()
Solution 3
The following code worked perfectly for me. Thanks to Hasan's answer.
from pynput.mouse import Listener
def is_clicked(x, y, button, pressed):
if pressed:
print('Clicked ! ') #in your case, you can move it to some other pos
return False # to stop the thread after click
with Listener(on_click=is_clicked) as listener:
listener.join()
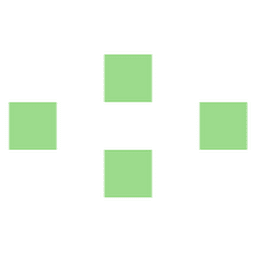
Comments
-
TheDetective about 3 years
so I'm trying to build a short script in Python. What I want to do is that if the mouse is clicked, the mouse will reset to some arbitrary position (right now the middle of the screen). I'd like this to run in the background, so it could work in in the OS (most likely Chrome, or some web browser). I'd also like it so that a user could hold down a certain button (say ctrl) and they could click away and not have the position reset. This way they could close the script without frustration.
I'm pretty sure I know how to do this, but I'm not sure as to what library to use. I'd prefer if it was cross platform, or at least Windows + Mac. Here's my code so far:
#! python3 # resetMouse.py - resets mouse on click - usuful for students with # cognitive disabilities. import pymouse width, height = m.screen_size() midWidth = (width + 1) / 2 midHeight = (height + 1) / 2 m = PyMouse() k = PyKeyboard() def onClick(): m.move(midWidth, midHeight) try: while True: # if button is held down: # continue # onClick() except KeyboardInterrupt: print('\nDone.')
-
FrankS101 over 5 yearsCan you also explain your answer? Only code is not a proper SO answer (from review)
-
exec85 almost 4 yearshow can i make it that when i release the button, it will go back "wait" mode ?