Python conversion from binary string to hexadecimal
Solution 1
int
given base 2 and then hex
:
>>> int('010110', 2)
22
>>> hex(int('010110', 2))
'0x16'
>>>
>>> hex(int('0000010010001101', 2))
'0x48d'
The doc of int
:
int(x[, base]) -> integer Convert a string or number to an integer, if possible. A floating
point argument will be truncated towards zero (this does not include a string representation of a floating point number!) When converting a string, use the optional base. It is an error to supply a base when converting a non-string. If base is zero, the proper base is guessed based on the string content. If the argument is outside the integer range a long object will be returned instead.
The doc of hex
:
hex(number) -> string Return the hexadecimal representation of an integer or long
integer.
Solution 2
bstr = '0000 0100 1000 1101'.replace(' ', '')
hstr = '%0*X' % ((len(bstr) + 3) // 4, int(bstr, 2))
Solution 3
Use python's binascii module
import binascii
binFile = open('somebinaryfile.exe','rb')
binaryData = binFile.read(8)
print binascii.hexlify(binaryData)
Solution 4
Converting Binary into hex without ignoring leading zeros:
You could use the format() built-in function like this:
"{0:0>4X}".format(int("0000010010001101", 2))
Solution 5
Using no messy concatenations and padding :
'{:0{width}x}'.format(int(temp,2), width=4)
Will give a hex representation with padding preserved
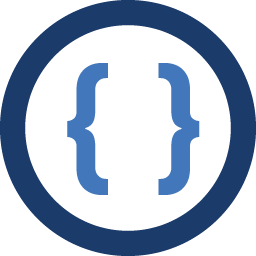
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
How can I perform a conversion of a binary string to the corresponding hex value in Python?
I have
0000 0100 1000 1101
and I want to get048D
I'm using Python 2.6. -
Ignacio Vazquez-Abrams over 14 yearsThis fails to preserve leading 0s.
-
SilentGhost over 14 yearsyou don't need list comprehension there
-
Eli Bendersky over 14 years@Ignacio, you're right, but I don't think the OP asked about that. In any case, ++ to your answer for pointing that out.
-
Eli Bendersky over 14 years@SO should really add per-language coloring. Here it thinks // is a C++ comment and grays out everything following. // in Python isn't a comment, but truncating integer division
-
John Machin over 14 years@Eli: the OP specifically said he wanted
048d
i.e. wants leading zero, DOESN'T want 0x -
ghostdog74 over 14 yearsso what's the problem? Its still in Python 2.6
-
John Machin over 14 yearsIt's still in 2.X for the benefit of people who were using it in 1.X. string.atoi() is according to the 2.6 docs """Deprecated since version 2.0: Use the int() built-in function.""" and is not present in 3.X. The 2.X implementation of string.atoi() calls int(). There is no good reason for telling some newcomer that string.atoi() even exists let alone telling them to use it instead of telling them to use int().
-
Andrei almost 11 yearsThis works only for 2-byte numbers, while Ignacio's answer works for any length.
-
Andrei almost 11 yearsYou need to replace spaces before conversion.
-
Andrei almost 11 yearsYou still need to replace spaces if any.
-
Andrei almost 11 yearsBrilliant answer. I am surprised why it's not upvoted/accepted yet.
-
Dennis over 10 yearsThis may not preserve the leading zeroes, but it's much more readable than the clever hacks below.
-
user253751 about 10 years@Dennis the first line is just cleaning up the binary string. The second line formats it as a hexadecimal string, padded to
(len(bstr) + 3) // 4
hex digits, which isnumber of bits / 4
rounded up, i.e. the number of hex digits required. The last part of the second line parses the binary string to a number, because the %X format specifier is for numbers not binary strings. -
Michal aka Miki almost 9 yearsWhere is bin_value_to_hex_string?
-
Djunzu over 6 yearsWhat is the equivalent in Python 3? Using "".format()