Python convert epoch time to day of the week
10,651
Solution 1
ep = 1412673904406
from datetime import datetime
print datetime.fromtimestamp(ep/1000).strftime("%A")
Tuesday
def ep_to_day(ep):
return datetime.fromtimestamp(ep/1000).strftime("%A")
Solution 2
import time
epoch = 1496482466
day = time.strftime('%A', time.localtime(epoch))
print day
>>> Saturday
Solution 3
from datetime import date
def convert_epoch_time_to_day_of_the_week(epoch_time_in_miliseconds):
d = date.fromtimestamp(epoch_time_in_miliseconds / 1000)
return d.strftime('%A')
Tested, returned Tuesday.
Solution 4
If you have milliseconds, you can use the time
module:
import time
time.strftime("%A", time.gmtime(epoch/1000))
It returns:
'Tuesday'
Note we use %A
as described in strftime:
time.strftime(format[, t])
%A Locale’s full weekday name.
As a function, let's convert the miliseconds to seconds:
import time
def convert_epoch_time_to_day_of_the_week(epoch_milliseconds):
epoch = epoch_milliseconds / 1000
return time.strftime("%A", time.gmtime(epoch))
Testing...
Today is:
$ date +"%s000"
1412674656000
Let's try another date:
$ date -d"7 Jan 1993" +"%s000"
726361200000
And we run the function with these values:
>>> convert_epoch_time_to_day_of_the_week(1412674656000)
'Tuesday'
>>> convert_epoch_time_to_day_of_the_week(726361200000)
'Wednesday'
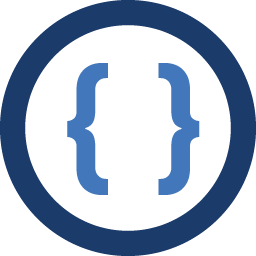
Author by
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
How can I do this in Python? I just want the day of the week to be returned.
>>> convert_epoch_time_to_day_of_the_week(epoch_time_in_miliseconds) >>> 'Tuesday'