Python CRC8 calculation
Solution 1
0x8a
corresponds to a standard CRC-8:
width=8 poly=0x07 init=0x00 refin=false refout=false xorout=0x00 check=0xf4 residue=0x00 name="CRC-8"
The Python crc8 you linked to will do exactly what you want.
For example (in Python 3):
hash.update(b'\x00\x11\x23\x32\x1C\xAC\x23\x3F\x25\x47\x3D\xB7\xE2\xC5\x6D\xB5\xDF\xFB\x48\xD2\xB0\x60\xD0\xF5\xA7\x10\x96\xE0\x00\x00\x00\x00\x00\x00\x00\x00\xC5')
print(hash.hexdigest())
gives:
8a
If you include the 8a
in the data, then the result is zero.
Solution 2
import crc8
hash = crc8.crc8()
hash.update("001123321CAC233F25473DB7E2C56DB5DFFB48D2B060D0F5A71096E00000000000000000C5".decode("hex"))
print( hash.hexdigest() )
Output:
8a
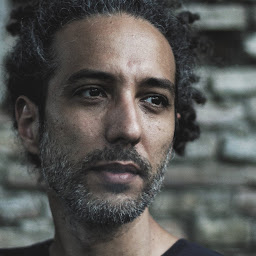
orestino
Updated on July 10, 2022Comments
-
orestino almost 2 years
I have some bytes I want to calculate the CRC8 on in python.
I don't have such an experience with that but I know, from the tech specs of my device, that this calculation has to be made with a 0x07 polynom, and a 0x00 initialization.
Let's take an use case. I've received this list of bytes where I know the last one is the CRC:
0x00 0x11 0x23 0x32 0x1C 0xAC 0x23 0x3F 0x25 0x47 0x3D 0xB7 0xE2 0xC5 0x6D 0xB5 0xDF 0xFB 0x48 0xD2 0xB0 0x60 0xD0 0xF5 0xA7 0x10 0x96 0xE0 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0xC5 0x8A
Now, how can I calculate the CRC my side in order to check if it corresponds to 0x8A?
I've made some research and tried different python modules like crcmod, crc8 and libscrc but I was not able to make them work: sometimes I've got a
MemoryError
error on the console!I've also tried with the following code but it doesn't seem to return me what I think is the correct CRC (0x8a):
import crc8 hash = crc8.crc8() hash.update("0x001123321CAC233F25473DB7E2C56DB5DFFB48D2B060D0F5A71096E00000000000000000C58A".encode('utf-8')) print( hash.hexdigest() )
What I'm doing wrong?
Is there anyone with some experience with who can help me? Maybe posting a snippet of code I can use to make the calculation?
However, any help will be appreciated! Thank you so much for your support...
-
orestino over 5 yearsThank you Mark, so I'm wondering why my code doesn't seem to return me the correct (at least I think it is) 0x8A CRC code. Here's the code: <code> import crc8 hash = crc8.crc8() hash.update("0x001123321CAC233F25473DB7E2C56DB5DFFB48D2B060D0F5A71096E00000000000000000C58A".encode('utf-8')) print( hash.hexdigest() ) </code> Is there somenthing I'm doing wrong?
-
Mark Adler over 5 yearsPut that code in your question. You seem to have a very basic misunderstanding of what a list of bytes actually is.
-
orestino over 5 yearsThank you Mark, I've placed the code in my question now. Yes I know, I have not such an experience in working with bytes in python. However, what do you mean with list of bytes? Do you means I have to use the
bytes
object? -
Mark Adler over 5 yearsWhat do you mean? You're the one who said "I've received this list of bytes". How did you receive it?
-
Mark Adler over 5 yearsThe CRC needs to be given the actual binary bytes. Not a hexadecimal representation of the bytes in a text string.