Python DFS and BFS
17,792
Solution 1
Yes, it is DFS.
To write a BFS you just need to keep a "todo" queue. You probably also want to turn the function into a generator because often a BFS is deliberately ended before it generates all possible paths. Thus this function can be used to be find_path or find_all_paths.
def paths(graph, start, end):
todo = [[start, [start]]]
while 0 < len(todo):
(node, path) = todo.pop(0)
for next_node in graph[node]:
if next_node in path:
continue
elif next_node == end:
yield path + [next_node]
else:
todo.append([next_node, path + [next_node]])
And an example of how to use it:
graph = {'A': ['B', 'C'],
'B': ['C', 'D'],
'C': ['D'],
'D': ['C'],
'E': ['F'],
'F': ['C']}
for path in paths(graph, 'A', 'D'):
print path
Solution 2
def recursive_dfs(graph, start, path=[]):
'''recursive depth first search from start'''
path=path+[start]
for node in graph[start]:
if not node in path:
path=recursive_dfs(graph, node, path)
return path
def iterative_dfs(graph, start, path=[]):
'''iterative depth first search from start'''
q=[start]
while q:
v=q.pop(0)
if v not in path:
path=path+[v]
q=graph[v]+q
return path
def iterative_bfs(graph, start, path=[]):
'''iterative breadth first search from start'''
q=[start]
while q:
v=q.pop(0)
if not v in path:
path=path+[v]
q=q+graph[v]
return path
'''
+---- A
| / \
| B--D--C
| \ | /
+---- E
'''
graph = {'A':['B','C'],'B':['D','E'],'C':['D','E'],'D':['E'],'E':['A']}
print 'recursive dfs ', recursive_dfs(graph, 'A')
print 'iterative dfs ', iterative_dfs(graph, 'A')
print 'iterative bfs ', iterative_bfs(graph, 'A')
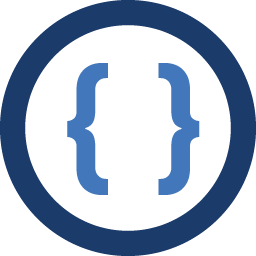
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Here http://www.python.org/doc/essays/graphs/ is DFS right ?
I try to do something with 'siblings', but it does not work. Can anyone write BFS similar to code from this site.