Python Email, [Errno 10061] No connection could be made because the target machine actively refused it
Solution 1
As mentioned by Cid-EL, You have to enable less secure apps in GMAIL settings
However, It's not secure to turn on that perticular option.
Gmail provides API's to perform mail operations. GMAIL API's are supported in many languages.
For Python API : Python with Gmail
For Java API's : Java with Gmail
Little more effort but you can have a secure connection !!
Step 1: Turn on the GMAIL API
Step 2: Install Google client library
pip install --upgrade google-api-python-client
Step 3: Code sample to send a mail using GMAIL API
from __future__ import print_function
import httplib2
import os
from apiclient import discovery
import oauth2client
from oauth2client import client
from oauth2client import tools
try:
import argparse
flags = argparse.ArgumentParser(parents=[tools.argparser]).parse_args()
except ImportError:
flags = None
# If modifying these scopes, delete your previously saved credentials
# at ~/.credentials/gmail-python-quickstart.json
SCOPES = "https://mail.google.com/"
CLIENT_SECRET_FILE = 'client_secret.json'
APPLICATION_NAME = 'Gmail API Python Quickstart'
def get_credentials():
"""Gets valid user credentials from storage.
If nothing has been stored, or if the stored credentials are invalid,
the OAuth2 flow is completed to obtain the new credentials.
Returns:
Credentials, the obtained credential.
"""
home_dir = os.path.expanduser('~')
credential_dir = os.path.join(home_dir, '.credentials')
if not os.path.exists(credential_dir):
os.makedirs(credential_dir)
credential_path = os.path.join(credential_dir,
'gmail-python-quickstart.json')
store = oauth2client.file.Storage(credential_path)
credentials = store.get()
if not credentials or credentials.invalid:
flow = client.flow_from_clientsecrets(CLIENT_SECRET_FILE, SCOPES)
flow.user_agent = APPLICATION_NAME
if flags:
credentials = tools.run_flow(flow, store, flags)
else: # Needed only for compatibility with Python 2.6
credentials = tools.run(flow, store)
print('Storing credentials to ' + credential_path)
return credentials
# create a message
def CreateMessage(sender, to, subject, message_text):
"""Create a message for an email.
Args:
sender: Email address of the sender.
to: Email address of the receiver.
subject: The subject of the email message.
message_text: The text of the email message.
Returns:
An object containing a base64 encoded email object.
"""
message = MIMEText(message_text)
message['to'] = to
message['from'] = sender
message['subject'] = subject
return {'raw': base64.b64encode(message.as_string())}
#send message
def SendMessage(service, user_id, message):
"""Send an email message.
Args:
service: Authorized Gmail API service instance.
user_id: User's email address. The special value "me"
can be used to indicate the authenticated user.
message: Message to be sent.
Returns:
Sent Message.
"""
try:
message = (service.users().messages().send(userId=user_id, body=message).execute())
#print 'Message Id: %s' % message['id']
return message
except errors.HttpError, error:
print ('An error occurred: %s' % error)
def main():
"""Shows basic usage of the Gmail API.
Send a mail using gmail API
"""
credentials = get_credentials()
http = credentials.authorize(httplib2.Http())
service = discovery.build('gmail', 'v1', http=http)
msg_body = "test message"
message = CreateMessage("[email protected]", "[email protected]", "Status report of opened tickets", msg_body)
SendMessage(service, "me", message)
if __name__ == '__main__':
main()
Solution 2
As far as I know Gmail and other companies have security to prevent emails being sent from unknown sources, but this link can turn that kind of security off.
There you can modify the account so it will accept the email.
I use this code to send email:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from_address = '[email protected]'
to_address = '[email protected]'
message = MIMEMultipart('Foobar')
epos_liggaam['Subject'] = 'Foobar'
message['From'] = from_address
message['To'] = to_address
content = MIMEText('Some message content', 'plain')
message.attach(content)
mail = smtplib.SMTP('smtp.gmail.com', 587)
mail.ehlo()
mail.starttls()
mail.login(from_address, 'password')
mail.sendmail(from_address,to_address, message.as_string())
mail.close()
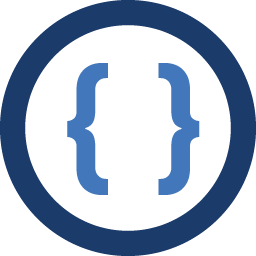
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I want to send a mail in using python, below is my code, but I got the error 10061 at the end, hope anyone can help me, thanks!
import smtplib fromaddr = '[email protected]' toaddrs = '[email protected] ' msg = 'Why,Oh why!' username = 'XXXXXX' password = 'XXXXXX' server = smtplib.SMTP('smtp.gmail.com:587') server.ehlo() server.starttls() server.login(username,password) server.sendmail(fromaddr, toaddrs, msg) server.quit()
error msg:socket.error: [Errno 10061] No connection could be made because the target machine actively refused it