Python ftplib - specify port
31,724
Solution 1
>>> from ftplib import FTP
>>> HOST = "localhost"
>>> PORT = 12345 # Set your desired port number
>>> ftp = FTP()
>>> ftp.connect(HOST, PORT)
Solution 2
After searching numerous solutions, a combination of the docs.python.org and the connect
command solved my issue.
from ftplib import FTP_TLS
host = 'host'
port = 12345
usr = 'user'
pwd = 'password'
ftps = FTP_TLS()
ftps.connect(host, port)
# Output: '220 Server ready for new user.'
ftps.login(usr, pwd)
# Output: '230 User usr logged in.'
ftps.prot_p()
# Output: '200 PROT command successful.'
ftp.nlst()
# Output: ['mysubdirectory', 'mydoc']
If you're using plain FTP instead of FTPS, just use ftplib.FTP
instead.
Solution 3
Yes you can use connect
from ftplib import FTP
my_ftp = FTP()
my_ftp.connect('localhost', 80) # 80 is the port for example
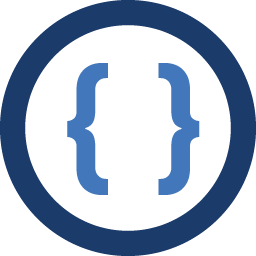
Author by
Admin
Updated on August 10, 2020Comments
-
Admin over 3 years
I would like to specify the port with Python's ftplib client (instead of default port 21).
Here is the code:
from ftplib import FTP ftp = FTP('localhost') # connect to host, default port
Is there an easy way to specify an alternative port?