Python: How to search an xml file for a list of elements then print them
You can iterate over children of a tag:
import xml.etree.ElementTree as ET
command = "ls"
switches = ["a", "l"]
fttkXML = ET.parse('fttk.xml') #parse the xml file into an elementtree
findCommand = fttkXML.find(command) #find the command in the elementtree
if findCommand != None:
print findCommand.text #prints that tag's text
for sub in list(findCommand): # find all children of command. In older versions use findCommand.getchildren()
if sub.tag in switches: # If child in switches
print sub.text # print child tag's text
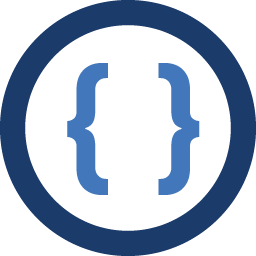
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have an xml file that I need to parse. It is below: It is called "fttk.xml"
<root> <ls> this is the ls <a> this is the ls -a </a> <l> this is the ls -l </l> </ls> <dd> this is the dd <a> this is the dd -a </a> <l> this is the dd -l </l> </dd> </root>
Fairly simple. I want to be able to print out the text in either "ls" or "dd" tags. Then print out the tags underneath them, if specified.
So far I've managed to be able to find the "ls" or "dd" tag in the XML and print out the text within the tag. I've done this with this code:
import xml.etree.ElementTree as ET command = "ls" fttkXML = ET.parse('fttk.xml') #parse the xml file into an elementtree findCommand = fttkXML.find(command) #find the command in the elementtree if findCommand != None: print (findCommand.text) #prints that tag's text
With that, I've saved off everything between "ls"..."/ls" tags. Now I want to search for the two flags ("a" and "l") beneath them, if specified, and print them. The tags are provided by a list like so:
switches = ["a", "l"]
However, I've tried to find something in ElementTree that will allow me to search for these tags from a list and print them out separately, however, the 'find' and 'findall' commands for an ElementTree or Element, returns "uhashable type list" when I attempt to feed it the 'switches' list.
So, how would I search for a list of tags and have the text printed out for each tag?
Thank you for your time.
Best Regards, J