Python: List of sublists, write sublists without brackets
Solution 1
First, the answer:
file.write(','.join(map(repr, List))
Your actual code can't possibly be writing what you claim it is, for multiple reasons:
- A file's
write
method will give you aTypeError
if you give it a list, not print it out. You have to callstr
orrepr
on the list to get something you canwrite
. - Calling
str
orrepr
on a list puts spaces after the commas, as well as brackets. - You've got at least one
SyntaxError
in your code that would prevent you even getting that far.
So, I have to guess what you're actually doing before I can explain how to fix it. Maybe something like this:
file.write(str(List))
If so, the __str__
method of a list is effectively:
'[' + ', '.join(map(repr, self)) + ']'
You want this without the brackets and without the spaces, so it's:
','.join(map(repr, self))
Solution 2
Your output format appears to be CSV. If the actual data is as simple as in the example provided, then you can just use python's csv writer:
import csv
with open('/tmp/myfile.txt', 'w') as f:
csv_writer = csv.writer(f)
csv_writer.writerows(List)
Solution 3
If you're on Python 3.x or 2.6 onwards and don't mind using print
as a function:
from __future__ import print_function # ignore if on py 3.x
for line in List:
print(*line, sep=',', file=file)
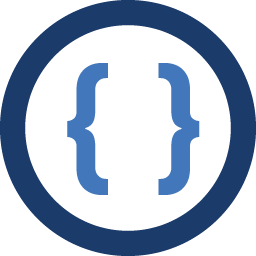
Admin
Updated on July 31, 2022Comments
-
Admin almost 2 years
I have a List that is x values long. The list is broken up into different length sublists.I then take these sublists and write them to an output file. Is there a way to print the sublists without the brackets.
Edited to clarfiy:
The actual list is 300 sublists long with 12250 elements in it, and changes with each senario I run. Obviously, this is simplified. Let me change a few things and see if that changes the answers.
i.e.
List=[[1, 2, 3], [4, 5, 6, 7], [8, 9, 10, 11, 12, 15]]
where the output is:
[1, 2, 3] [4, 5, 6, 7] [8, 9, 10, 11, 12, 15]
but I want
1, 2, 3 4, 5, 6, 7 8, 9, 10, 11, 12, 15
I want this output in a new variable, say ListMod, so then I can use ListMod in a seperate function to write a file. The file also calls 2 other lists, Time and Size, where Size designates the length of the line in ListMod.
How much does that modify your answers?