Python: pygame.QUIT()
19,345
Solution 1
Calling sys.exit()
raises the SystemExit
exception, so that's perfectly normal.
For example, try changing your exit call to sys.exit(1)
and you will see the new exit code reflected in your traceback:
Traceback (most recent call last):
File "C:/.../pygame.py", line 8, in <module>
sys.exit(1);
SystemExit: 1
In other news, you probably don't need to be explicitly calling pygame.quit()
- the documentation suggests letting your program quit in the normal way.
Solution 2
Toggle a bool when you hit escape. Then you can cleanly save data other in places, if needed.
done = False
while not done:
#events
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
draw()
Solution 3
I recalled four events for a simple music player in python. The code here below
import pygame
from pygame import mixer
pygame.init()
mixer.init()
screen = pygame.display.set_mode((600, 400))
mixer.music.load("song.mp3")
mixer.music.play()
print("Press 'p' to pause 'r' to resume")
print("Press 'q' to quit")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_s:
mixer.music.stop()
if event.key == pygame.K_r:
mixer.music.play()
if event.key == pygame.K_p:
mixer.music.pause()
if event.key == pygame.K_y:
mixer.music.unpause()
pygame.QUIT()
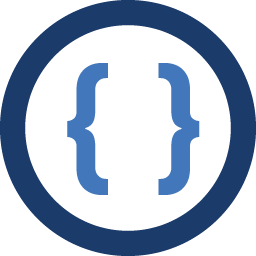
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Just been messing around with pygame and ran into this error.
CODE:
import sys import pygame pygame.init() size = width, height = 600, 400 screen = pygame.display.set_mode(size) while 1: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit(); sys.exit();
ERROR:
Traceback (most recent call last): File "C:/Users/Mike Stamets/Desktop/Mygame/Pygame practice/ScreenPractice.py", line 12, in <module> pygame.quit(); sys.exit(); SystemExit
I was just trying to set a while loop so that when the red X is pressed then the program will exit. What is going wrong?