Python raw_input ignore newline
Solution 1
Because raw_input
only takes one line from input you need to create a loop:
names = []
print('Shoot me some names partner: ')
while True:
try:
name = raw_input()
except KeyboardInterrupt:
break
names.append(name)
print('What do you want to do?')
print('1 - format names for program 1')
print('2 - format names for program 2')
first_act = raw_input('Enter choice: ')
print(names)
print(first_act)
Test run:
Shoot me some names partner:
name1
name2
^CWhat do you want to do?
1 - format names for program 1
2 - format names for program 2
Enter choice: 1
['name1', 'name2']
1
Note I've used ^C
(Ctrl-C) here to indicate the end of the input.
Solution 2
I am not sure what you are trying to ask but when you use raw_input(), it strips a trailing newline.
And the doc says the same too.
If the prompt argument is present, it is written to standard output without a trailing newline. The function then reads a line from input, converts it to a string (stripping a trailing newline), and returns that. When EOF is read, EOFError is raised.
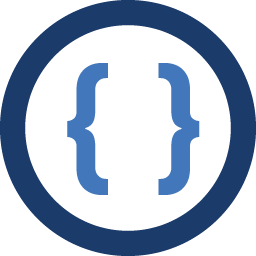
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
Is there a way to ignore newline characters in data entered through raw_input? I am trying to use raw_input to input a list of strings that are copied and pasted from a spreadsheet. the problem is that it appears that the new line characters cause the data to be entered prematurely. All the empty spaces will be stripped anyway, so removing the newlines as the data is entered would be an added benefit. This data needs to be entered directly through the terminal prompt and not read from a file.
This is what I have done so far:
names = raw_input('Shoot me some names partner: ') print 'What do you want to do?' print '1 - format names for program 1' print '2 - format names for program 2' first_act = raw_input('Enter choice: ') print names print first_act
Now when I run this and input the dummy names I put into a google doc spreadsheet to test, as soon as I hit shift+ctl+v, without hitting enter I get this:
seth@linux-1337:~> python pythonproj/names.py Shoot me some names partner: abcd,efg,hijkl,mnop abcd,efg,hijkl,mnop abcd,efg,hijkl,mnop abcd,efg,hijkl,mnop abcd,efg,hijkl,mnop abcd,efg,hijkl,mnop abcd,efg,hijkl,mnop abcd,efg,hijkl,mnopWhat do you want to do? 1 - format names for program 1 2 - format names for program 2 Enter choice: abcd,efg,hijkl,mnop abcd,efg,hijkl,mnop seth@linux-1337:~> abcd,efg,hijkl,mnop If 'abcd,efg,hijkl,mnop' is not a typo you can use command-not-found to lookup the package that contains it, like this: cnf abcd,efg,hijkl,mnop seth@linux-1337:~> abcd,efg,hijkl,mnop If 'abcd,efg,hijkl,mnop' is not a typo you can use command-not-found to lookup the package that contains it, like this: cnf abcd,efg,hijkl,mnop seth@linux-1337:~> abcd,efg,hijkl,mnop If 'abcd,efg,hijkl,mnop' is not a typo you can use command-not-found to lookup the package that contains it, like this: cnf abcd,efg,hijkl,mnop seth@linux-1337:~> abcd,efg,hijkl,mnop If 'abcd,efg,hijkl,mnop' is not a typo you can use command-not-found to lookup the package that contains it, like this: cnf abcd,efg,hijkl,mnop seth@linux-1337:~> abcd,efg,hijkl,mnop If 'abcd,efg,hijkl,mnop' is not a typo you can use command-not-found to lookup the package that contains it, like this: cnf abcd,efg,hijkl,mnop seth@linux-1337:~> abcd,efg,hijkl,mnop
I am pretty new to python, and I am not the most experienced programmer by far. This is python 2.7.
-
Jay Wong over 8 yearsHi, how should I tell python I have finished my input? I tried
^c
, but it did not work.